The andThen Function in Scala
Mohammad Irfan
Mar 11, 2022
Scala
Scala Function
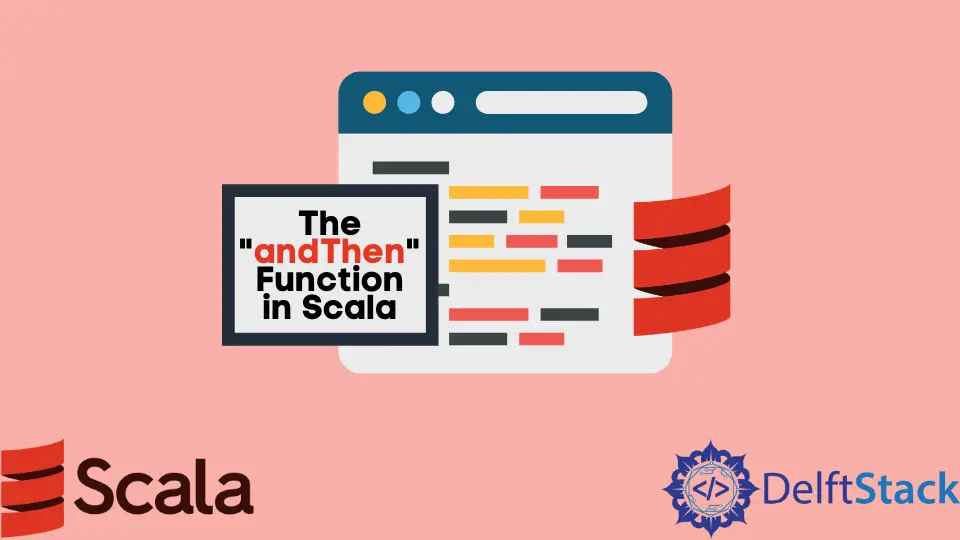
This tutorial will discuss the andThen
composition in Scala. Scala’s andThen
feature allows it to call functions in a composition manner.
If you want to call a function using the output instance, you can use this feature. You can also use it to call multiple functions in a chain sequence.
Use andThen
to Call Functions in Scala
We have two functions and call them using the andThen
. We called the first add
function and then the mul(multiply)
function.
object MyClass {
val add=(a: Int)=> {
a + 1
}
val mul=(a: Int)=> {
a * 2
}
def main(args: Array[String]) {
println((add andThen mul)(2))
}
}
Output:
6
The result above is based on the sequence of functions execution.
Let’s see another example of andThen
. We called two functions with different patterns/parameters in the following code snippet and got the desired result.
val incrementByTen : Int => Int = a => a+10
val multiplyByFive : Int => Int = num => num * 5
val result = incrementByTen.andThen(multiplyByFive)(10)
print("result "+result)
Output:
result 100
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe