How to Format Strings in Scala
- Creating a String in Scala
-
String Formatting Using the
s
Placeholder in Scala -
String Formatting Using the
f
Placeholder in Scala -
String Formatting Using the
raw
Placeholder in Scala -
String Formatting Using the
format()
Function in Scala
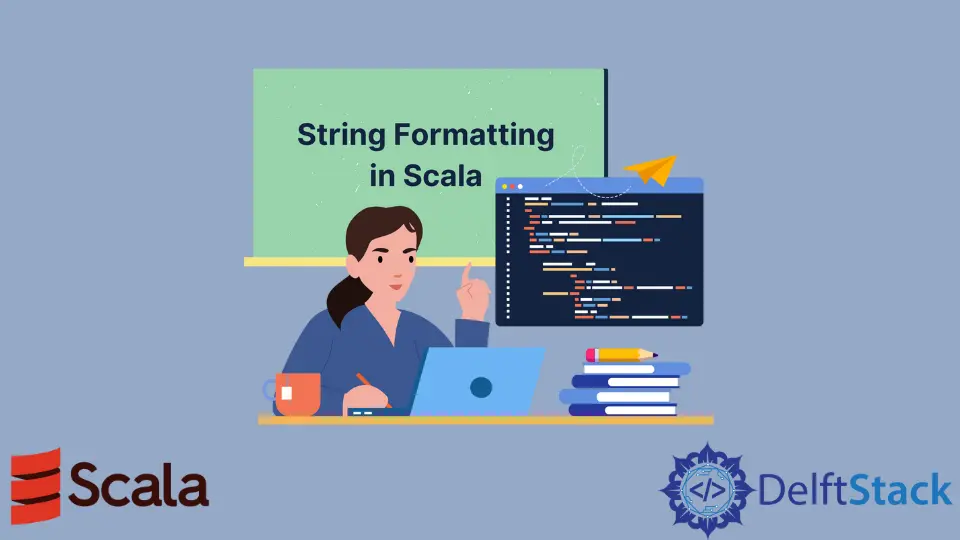
In this tutorial, we will discuss the string formatting procedure in Scala.
A string is a sequence of characters and is an immutable data type as in Java. We can create a string using string literals and append (join) using the plus (+
) operator.
After creating it, Scala provides several ways to format a string to make it more readable. In Scala, we can format the string using string interpolation.
Creating a String in Scala
We create a string by assigning it to a variable and printing it.
object MyClass {
def main(args: Array[String]) {
var str = "Scala String"
print(str)
}
}
Output:
Scala String
As we have already mentioned, a string is immutable, and modifying the string does not alter the original string. Here, we created a string and then modified it by adding a new string using the plus (+
) operator.
object MyClass {
def main(args: Array[String]) {
var str = "Scala String"
"Hello "+str
print(str)
}
}
Output:
Scala String
String Formatting Using the s
Placeholder in Scala
String interpolation is the process of formatting string in Scala. It provides some keys/placeholders that we can use with the string.
We used the s
placeholder with the string that allows to use of variables with the string and replace with the values during execution.
object MyClass {
def main(args: Array[String]) {
var a = 10
var b = 20.5;
println(s"value of a = $a")
println(s"value of b = $b")
}
}
Output:
value of a = 10
value of b = 20.5
String Formatting Using the f
Placeholder in Scala
We used the f
with the string that allows to use of variables with the string and replace with the values during execution. The variable must include $
with the variable.
object MyClass {
def main(args: Array[String]) {
var a = 10
var b = 20.5;
println(f"value of a = $a")
println(f"value of b = $b")
}
}
Output:
value of a = 10
value of b = 20.5
String Formatting Using the raw
Placeholder in Scala
If you want to print a raw string that includes all the formatting symbols, then use the raw
placeholder as a prefix with the string. You can clearly understand the difference between printing strings with or without this placeholder in the output.
object MyClass {
def main(args: Array[String]) {
var a = "Scala \tstring \n formatting"
var b = raw"Scala \tstring \n formatting"
println(a)
println(b)
}
}
Output:
Scala string
formatting
Scala \tstring \n formatting
String Formatting Using the format()
Function in Scala
Scala provides a string function format()
which acts as a print()
function of C. It is used to create a formatted string that you can use anywhere in your code.
object MyClass {
def main(args: Array[String]) {
var a = 1
var b = 10
var str1 = "This is %s string".format(a)
var str2 = "This is %s string".format(b)
println(str1)
println(str2)
}
}
Output:
This is 1 string
This is 10 string