Simple Build Tool in Scala
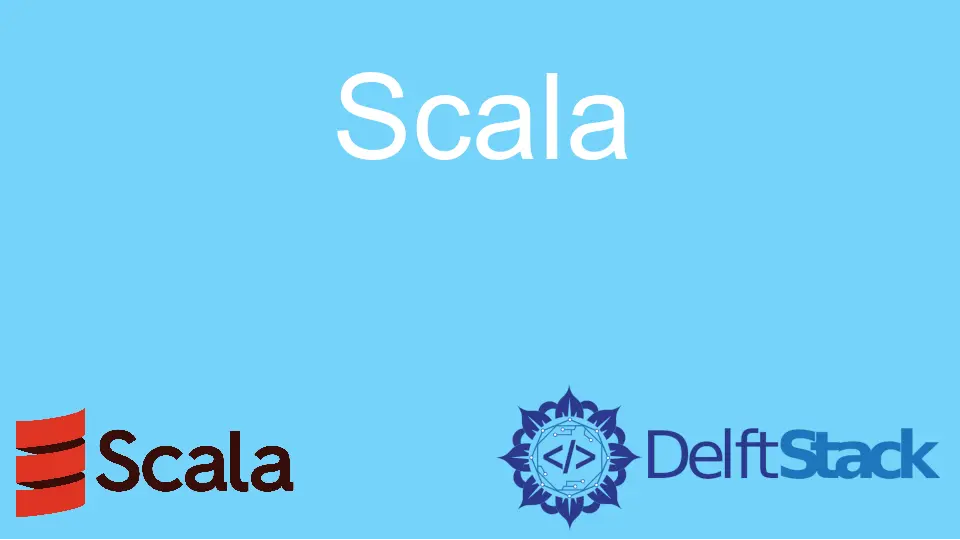
Simple Build Tool
, popularly known as SBT
, is an open-source build software used for Scala and Java projects.
Ant
and Maven
are well-known build tools. SBT
is the de facto build tool used in the Scala community.
Why Do We Need SBT
in Scala
The projects are very big and contain hundreds or thousands of source code in the real world. It becomes very tedious to compile all these source code files manually.
So we need a tool that can manage the compilation of all these files. That is where SBT
comes into the picture.
How Does SBT
Work in Scala
To create an SBT
project file, we will use build.sbt
. This file will list all the source files our project consists of, along with other information about our project.
Simple Build Tool
will read the file and compile it to complete the project.
Features in Simple Build Tool
are:
SBT
supports mixed Java and Scala code.SBT
does incremental testing and compilation, which means only the changed part in the source code is re-compiled, only affected tests are re-run.SBT
automatically buildsdependencies
.If we want to use some third-party or external libraries in our project, we automatically download the right versions of those libraries and include them in our project.SBT
is integrated with Scala interpreter for faster debugging and iteration.- Native support for compiling Scala code and integrating with many Scala test frameworks.