Sealed Traits in Scala
Mohammad Irfan
Apr 21, 2022
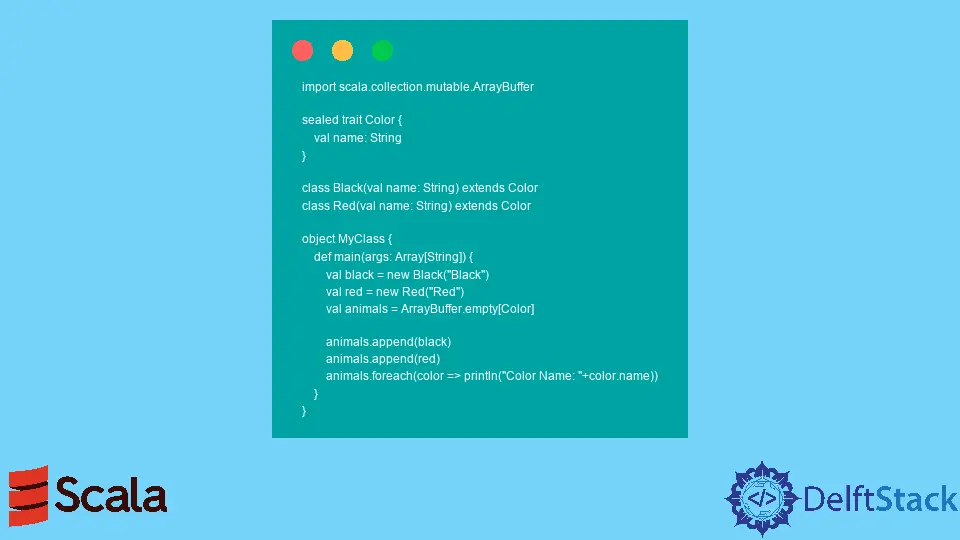
This tutorial will discuss the sealed traits with running examples in Scala.
Sealed Traits in Scala
Traits in Scala are similar to the interfaces of Java and are used to share data between classes. We can instantiate class, but traits cannot.
In Scala, traits can be marked as sealed, similar to classes, to restrict the inheritance. Sealed traits and all subtypes must be declared in the same file to ensure that all subtypes are known.
To define a trait, we use the trait
keyword, like:
trait Runnable
Similarly, to create sealed traits, we use the sealed
class, like:
sealed trait Runnable
Key points:
- A sealed trait can only be extended in the same file.
- The compiler easily knows all possible subtypes.
- It is recommended to use sealed traits only if the number of possible subtypes is finite and known in advance.
Create a Sealed Trait in Scala
Let’s understand by creating a sealed trait in Scala.
import scala.collection.mutable.ArrayBuffer
sealed trait Color {
val name: String
}
class Black(val name: String) extends Color
class Red(val name: String) extends Color
object MyClass {
def main(args: Array[String]) {
val black = new Black("Black")
val red = new Red("Red")
val animals = ArrayBuffer.empty[Color]
animals.append(black)
animals.append(red)
animals.foreach(color => println("Color Name: "+color.name))
}
}
Output:
Color Name: Black
Color Name: Red