Scala substring() Function
-
String
substring(int start_index)
in Scala -
String
substring(int starting_Index, int end_index)
in Scala - Conclusion
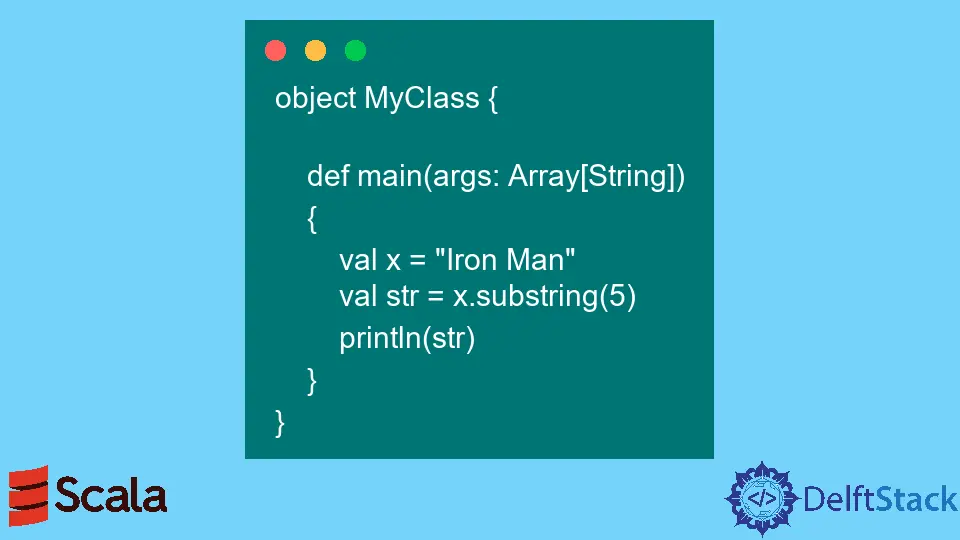
This article will teach about the substring
function in Scala.
As the name suggests, the inbuilt Scala substring
method is used to get the substring from the given input string. In Scala, we have two different variations of this method and based on our requirement; we can use any one among them.
Syntax:
substring(int index)
This is the basic method signature as per Scala doc, which we use; as we can see above, we have to pass the start index from where we want to fetch the content from the main string. Now let’s see the two variations of this.
String substring(int start_index)
in Scala
We have to pass just one parameter in this method, the start_index
. This parameter specifies the starting index so that the method can return the substring starting from the index we have specified.
Let’s see an example to understand it better.
object MyClass {
def main(args: Array[String])
{
val x = "Iron Man"
val str = x.substring(5)
println(str)
}
}
Output:
Man
We have created a string, Iron Man
, in the above code and stored it in the variable x
. I
is stored at index 0, r
is stored at index 1, o
is stored at index 2, etc.
We use the substring
method with parameter 5
; this will return the string starting from index 5. So the result will be Man
.
String substring(int starting_Index, int end_index)
in Scala
This method passes two parameters, starting_Index
and end_index
. This method is nothing but just an overloaded version of the normal version.
This returns the substring between the given range of indices.
Now let’s understand the parameter in a little depth.
starting_index
- This parameter is the same as discussed above.end_index
- This parameter is used to specify the end index of the substring. It specifies the endpoint of our substring where our substring will end, beginning fromstart_index
and finishing atend_index
.
Let’s see an example to understand it better.
object MyClass
{
def main(args: Array[String])
{
val x = "This is Tony stark"
val res = x.substring(5,16)
println(res)
}
}
Output:
is Tony sta
Conclusion
In this article, we learned about Scala’s substring
method, which returns the substring between the given range of indices. This inbuilt method in Scala is quite useful while performing string operations.