How to Sort a List in Scala
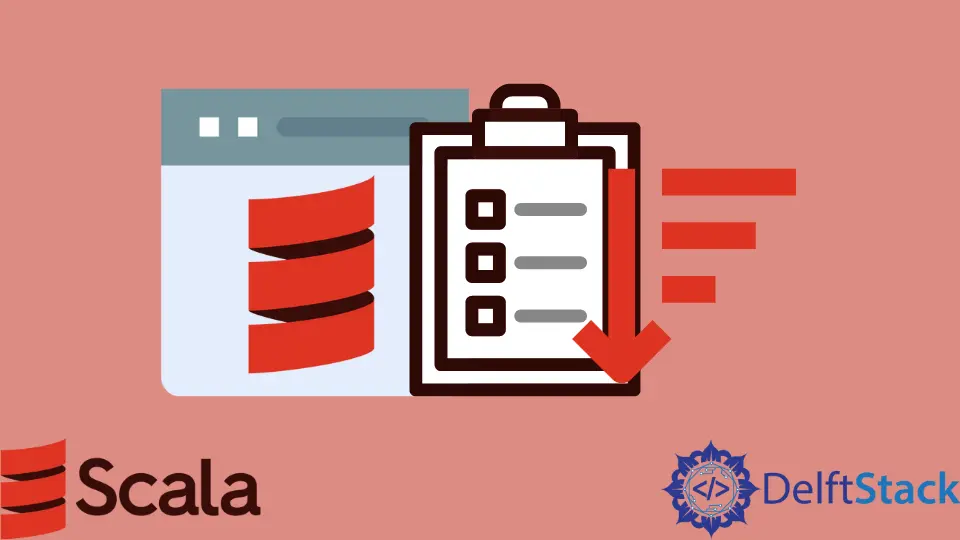
This tutorial will discuss the procedure to sort the list elements in Scala.
A list is a container used to store elements in linear order. There is a class List
in Scala to implement the list.
It provides several built-in functions to support its operations, such as inserting or deleting elements. Here, we will learn to sort the elements of a list in Scala.
Use Sorted Property to Sort List in Scala
We used the sorted property to sort the list elements in this example. First, we created a list with some elements and applied the property.
We also used the for
loop to traverse the elements to see the sorted elements.
object MyClass {
def main(args: Array[String]) {
var list1 = List("j", "b", "c")
val sorted = list1.sorted
for (name <- sorted) println(name)
}
}
Output:
b
c
j
Use the sortWith()
Function to Sort List in Scala
We used the sortWith()
function to take a lambda expression as an argument and return the sorted result. We can use this function to sort the list in ascending and descending order.
See the example below.
object MyClass {
def main(args: Array[String]) {
var list1 = List("j", "b", "c")
val sorted = list1.sortWith((a: String, b: String)
=> a.charAt(0).toLower < b.charAt(0).toLower)
println("--------ascending order-----------")
for (name <- sorted) println(name)
val sorted2 = list1.sortWith((a: String, b: String)
=> a.charAt(0).toLower > b.charAt(0).toLower)
println("--------descending order----------")
for (name <- sorted2) println(name)
}
}
Output:
--------ascending order-----------
b
c
j
--------descending order----------
j
c
b
We can also use the _
(underscore) to replace the redundant variables and make the code concise for readability. See the example below.
object MyClass {
def main(args: Array[String]) {
var list1 = List("mac", "apple", "dell")
val sorted = list1.sortWith(_.length < _.length)
println("--------ascending order-----------")
for (name <- sorted) println(name)
}
}
Output:
--------ascending order-----------
mac
dell
apple