How to Reassignment to a Val in Scala
- Understanding the Immutability of Val
- Using Mutable Variables
- Using Collections for State Management
- Utilizing Higher-Order Functions
- Conclusion
- FAQ
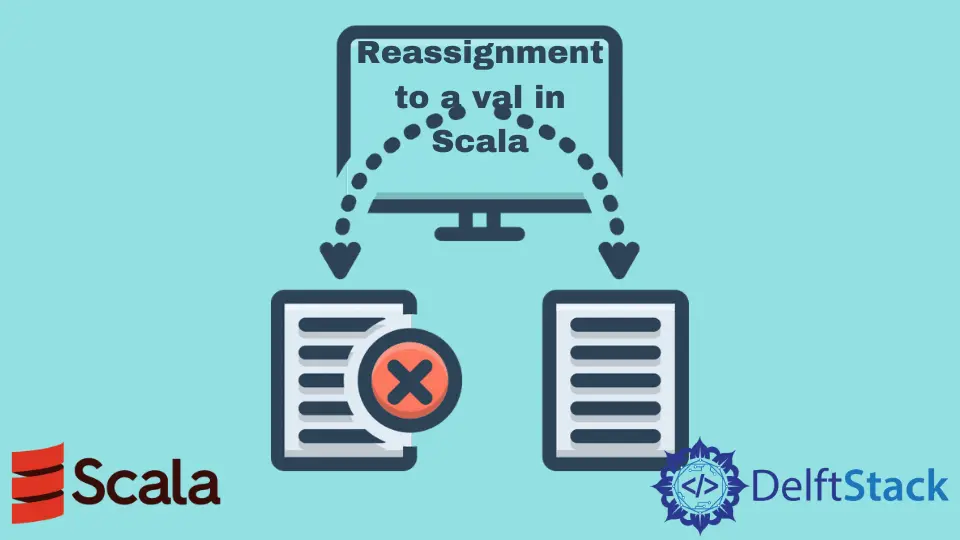
Reassigning a value to a val
in Scala can be a bit tricky, especially for those new to the language. In Scala, val
is used to declare constants, meaning once a value is assigned, it cannot be changed. This immutability is a core feature of Scala, promoting safer and more predictable code. However, there are scenarios where you might want to achieve a similar effect as reassignment.
In this article, we will explore various methods to work around the immutability of val
, focusing on effective strategies for managing state in your Scala applications. Whether you’re a beginner or an experienced developer, understanding these techniques will enhance your coding skills and enable you to write cleaner, more efficient Scala code.
Understanding the Immutability of Val
In Scala, when you declare a variable using val
, you create a read-only reference. This means that once assigned, the value cannot be changed. For example:
val number = 10
In this case, number
is a constant with a value of 10. Attempting to reassign it later in the code will lead to a compilation error. This is a fundamental aspect of functional programming, which Scala embraces. The immutability of val
helps prevent side effects and makes your code easier to reason about.
However, there are ways to work with mutable state in Scala while still adhering to the principles of immutability. Let’s explore some alternative methods that allow you to manage values effectively.
Using Mutable Variables
One of the simplest ways to work around the immutability of val
is by using a mutable variable instead. In Scala, you can declare a variable using var
, which allows for reassignment. Here’s how it works:
var mutableNumber = 10
mutableNumber = 20
Output:
20
In this example, mutableNumber
is initially set to 10, but later, it is reassigned to 20. This is straightforward and allows for flexibility in your code. However, using mutable variables can introduce side effects, which is why it’s essential to use them judiciously. If you find yourself needing to change values frequently, consider whether your design could be improved by embracing immutability instead.
When using var
, it’s crucial to maintain clarity in your code. Make sure to document why a variable needs to be mutable and ensure that its mutability is contained to a small scope. This helps maintain the overall integrity of your program.
Using Collections for State Management
Another effective method to manage state in Scala without directly reassigning a val
is to use collections. Collections in Scala, such as lists or maps, can hold multiple values, and you can create new collections based on existing ones. This approach maintains immutability while allowing you to “change” the data structure by creating a new instance.
Here’s an example using a list:
val numbers = List(1, 2, 3)
val updatedNumbers = numbers :+ 4
Output:
List(1, 2, 3, 4)
In this code snippet, we start with a list of numbers. Instead of changing the original list, we create a new list called updatedNumbers
that includes the new value. The original numbers
list remains unchanged. This technique encourages a functional programming style, where data is transformed rather than modified.
Using collections effectively can lead to cleaner code and easier debugging. You can also leverage Scala’s powerful collection methods to perform operations like filtering, mapping, and reducing, which can help manage state in a more functional way.
Utilizing Higher-Order Functions
Higher-order functions are a powerful feature in Scala that can help you manage state without the need for reassignment. These functions can take other functions as parameters or return them as results. By leveraging higher-order functions, you can encapsulate behavior and maintain immutability.
Here’s a simple example using a higher-order function:
def updateValue(value: Int, updater: Int => Int): Int = {
updater(value)
}
val increment = (x: Int) => x + 1
val newValue = updateValue(10, increment)
Output:
11
In this example, we define a function updateValue
that takes an integer and a function to update that integer. We then create an increment function and pass it to updateValue
. The result is a new value, demonstrating how you can effectively manage state without directly reassigning a val
.
Higher-order functions promote code reusability and can lead to more concise and expressive code. They allow you to abstract away the details of state management, focusing instead on the transformation of data.
Conclusion
Reassigning a value to a val
in Scala is not possible due to its immutable nature. However, by utilizing mutable variables, collections, and higher-order functions, you can effectively manage state in your applications. Embracing immutability can lead to safer, more predictable code, while these techniques allow you to achieve the flexibility you may need. As you continue to work with Scala, keep these strategies in mind to enhance your coding practices and improve your overall development experience.
FAQ
-
Can you reassign a val in Scala?
No, a val in Scala is immutable, meaning it cannot be reassigned once it has been initialized. -
What is the difference between val and var in Scala?
Val is immutable (read-only), while var is mutable (can be reassigned). -
How can I manage state without reassigning a val?
You can use mutable variables, collections, or higher-order functions to manage state effectively. -
Are there best practices for using mutable variables in Scala?
Yes, use mutable variables sparingly and document their purpose to maintain code clarity. -
Why is immutability important in functional programming?
Immutability helps prevent side effects, making code easier to reason about and less prone to bugs.