How to Print Case Classes Like Pretty Printed Tree in Scala
Suraj P
Feb 02, 2024
Scala
Scala Print
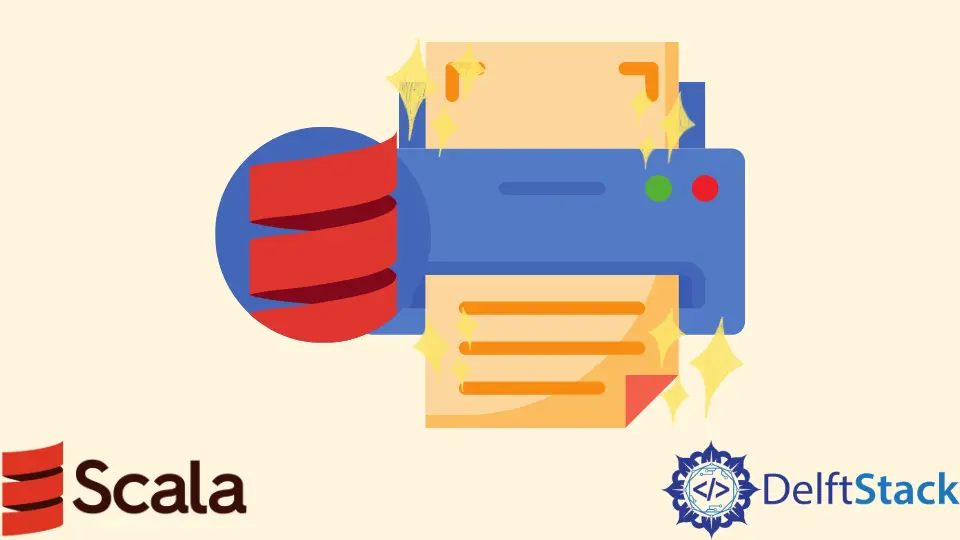
This article will teach you how to print case classes like (pretty-printed) trees in Scala.
Print Case Classes Like Pretty Printed Tree in Scala
Printing the output in a tree-like fashion is highly useful when making parsers in Scala.
We have a package called sext
that we can use to pretty print the case classes. It provides many useful functions, such as treeString
and valueTreeString
, which can print the output in a tree-like fashion.
But to make it work, we have to add a dependency in our library dependencies first. First, we have to open SBT dependency in our project and add the following line to it:
libraryDependencies += "com.github.nikita-volkov" % "sext" % "0.2.4"
Now we can use the import statement import sext._
in our program. Let us see an example to understand it better.
Example code:
object Example extends App {
import sext._
case class ourClass( kind : Kind, list : List[ tree ] )
sealed trait Kind
case object Complex extends Kind
case class tree( a : Int, b : String )
val store = ourClass(Complex,List(tree(1, "abcd"), tree(2, "efgh")))
println("output using treeString:\n")
println(store.treeString)
println()
println("output using valueTreeString:\n")
println(store.valueTreeString)
}
Output:
output using treeString:
ourClass:
- Complex
- List:
| - tree:
| | - 1
| | - abcd
| - tree:
| | - 2
| | - efgh
output using valueTreeString:
- kind:
- list:
| - - a:
| | | 1
| | - b:
| | | abcd
| - - a:
| | | 2
| | - b:
| | | efgh
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe