How to Convert Int to String in Scala
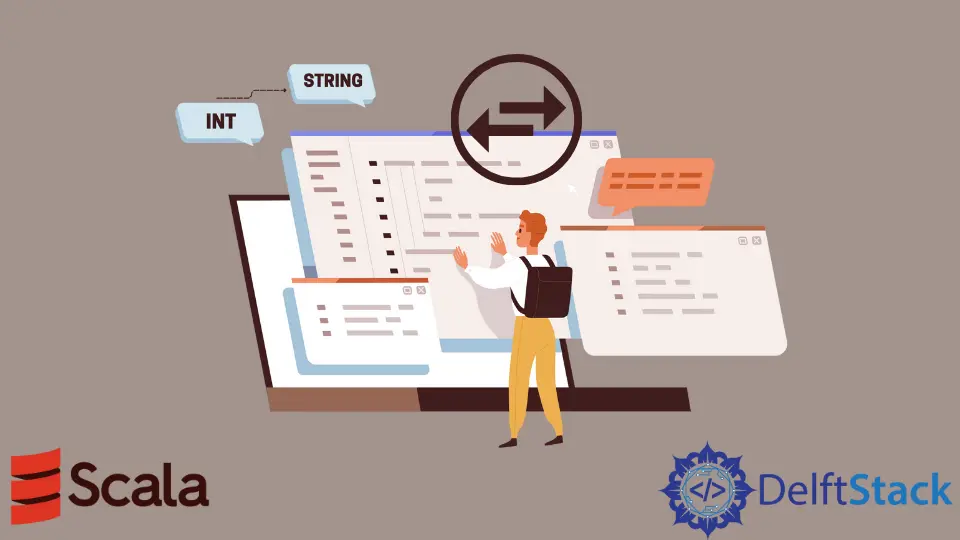
This article will teach how to convert an integer to a string in Scala.
Use the toString()
Method in Scala
In Scala, the toString()
method returns the string representation of the specified value.
Method definition:
def toString():String
Return type: This method returns the string representation of the value.
Syntax:
int_value.toString
Example code:
object MyClass {
def main(args: Array[String]) {
val ans = (1234).toString
println(ans)
println(ans.getClass)
}
}
Output:
1234
class java.lang.String
We have converted the integer 1234
to string using the toString
method and stored it in a variable ans
. Then we printed the answer along its class using the getClass
method.
Use the valueOf()
Method of Java
valueOf()
method converts the data into a string. It’s an overloaded static method for all Java’s built-in types within a string.
We can use this same method in our Scala programs as well.
Syntax:
String.valueOf(x)
It returns the string representation of integer x
.
Example code:
object MyClass {
def main(args: Array[String]) {
val num = 1234
val ans = String.valueOf(num)
println(ans)
println(ans.getClass)
}
}
Output:
1234
class java.lang.String
Use StringBuilder
in Scala
We know that String
objects are immutable, meaning they cannot be updated once created. We sometimes want the string to be modified frequently; we can use the StringBuilder
class in such cases.
This is very useful if we concatenate multiple values into a string.
Example code:
object MyClass {
def main(args: Array[String]) {
val x = 1234
val y = 567
val z = 900
val ans = new StringBuilder();
ans.append(x)
ans.append(y)
ans.append(z)
println(ans)
println(ans.getClass)
}
}
Output:
1234567900
class scala.collection.mutable.StringBuilder
The above code can be written using the StringBuffer
class; the StringBuffer
class is more thread-safe than the StringBuilder
class.