How to Concatenate Strings in Scala
-
Use the
concat()
Method to Concatenate Strings in Scala -
Use the
+
Operator to Concatenate Strings in Scala -
Use the
mkString
Method to Concatenate Strings in Scala
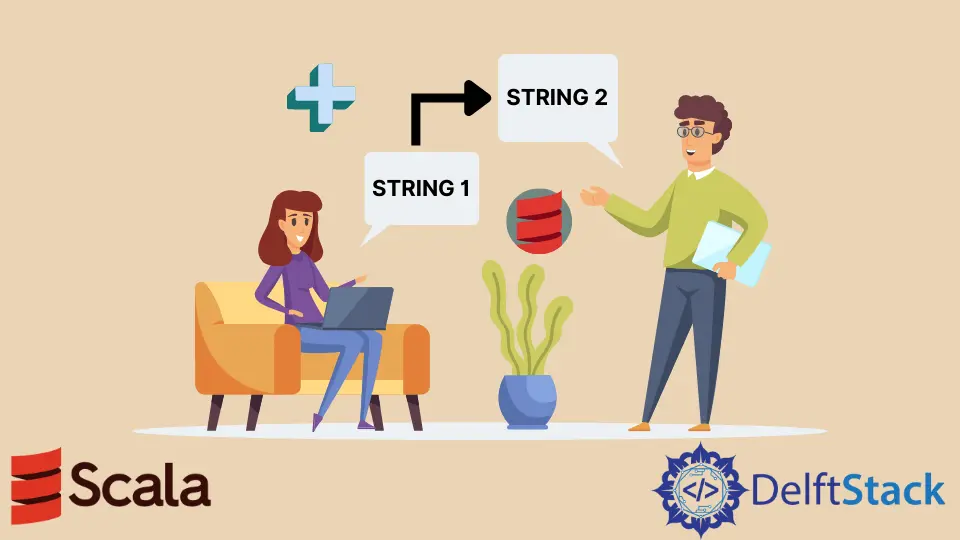
In this article, we will learn how to do concatenation of strings in Scala.
In Scala, string objects are immutable, meaning they are constant and cannot be updated or changed once created. Concatenation refers to creating a new string by adding two or more strings together.
We have different methods in Scala for concatenating strings; let’s look at them individually.
Use the concat()
Method to Concatenate Strings in Scala
Syntax:
val result = string_one.concat(string_two)
The concat()
method concats the string_two
at the end of string_one
and returns a new string which is a concatenation of both the strings.
Example code:
object MyClass {
def main(args: Array[String]) {
val str1 = "chicken"
val str2 = "nuggets"
var result = str1.concat(str2);
println("String 1:" +str1);
println("String 2:" +str2);
println("New String :" +result);
}
}
Output:
String 1:chicken
String 2:nuggets
New String :chickennuggets
Use the +
Operator to Concatenate Strings in Scala
Syntax:
val result = string_one + string_two + string_three ...
This method is more useful when we want to concat multiple strings.
Example code:
object MyClass {
def main(args: Array[String]) {
val str1 = "chicken"
val str2 = " nuggets"
val str3 = " are tasty "
var result = str1 + str2 + str3
println("New String :" +result);
}
}
Output:
New String :chicken nuggets are tasty
Sometimes we have to concat the strings present inside a collection. Let’s look at the methods to do so.
Use the mkString
Method to Concatenate Strings in Scala
mkString
is the most simple and idiomatic method that concatenates the collection elements.
Example code:
object MyClass {
def main(args: Array[String]) {
val str = List("chicken "," nuggets ","are tasty")
val result = str.mkString
println(result)
}
}
Output:
chicken nuggets are tasty
One advantage of this method is that we can also add a custom separator.
Example code:
object MyClass {
def main(args: Array[String]) {
val str = List("chicken "," nuggets ","are tasty")
val result = str.mkString(",")
println(result)
}
}
Output:
chicken , nuggets ,are tasty
In this article, we learned many different methods to join strings or strings present in a collection using Scala.