How to Return Boolean With If-Else in Scala
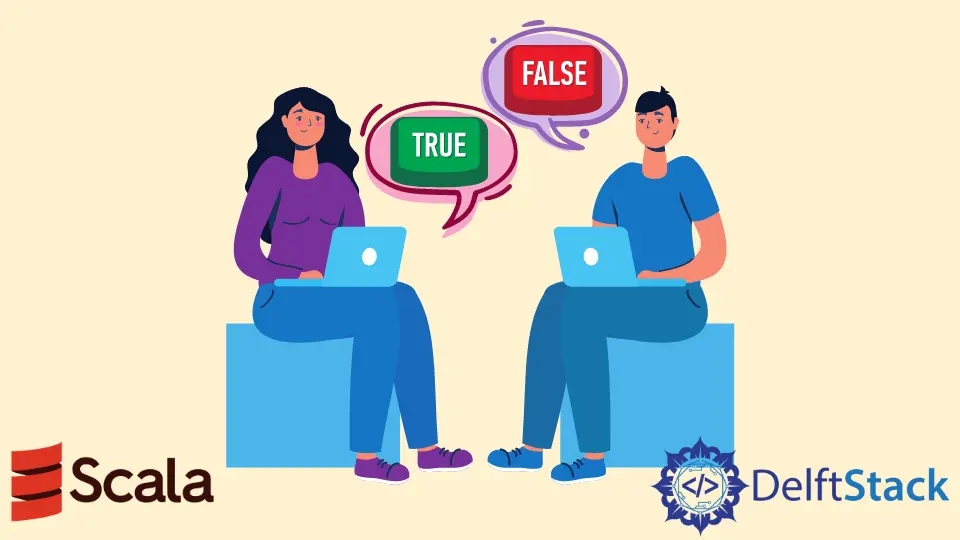
This article will teach us how to return Boolean values when working with if-else
in Scala.
Return Boolean With if-else
in Scala
Let’s see a scenario to understand it better.
def check(): Boolean = {
for ((digit1,digit2,digit3) <- digitsSet){
if ((1,5,6) == (digit1,digit2,digit3))
true
else
false
}
}
val digitsSet = Set((10,20,30),(1,5,6),(78,109,23),(14,25,57))
In the above code, we are trying to find whether our set contains those three digits, so we expect either true
or false
as the output, but when the above code is executed, we get the following error.
type mismatch;
found : Unit
required: Boolean
for ((digit1,digit2,digit3) <- digitsSet){
The problem is that the function should return Boolean instead of Unit
.
So we can solve this problem in different ways.
-
Using the
contains
method to find an element in our set. It returnstrue
if the element is present; else returnsfalse
.This is a more elegant way of writing the above code.
Example code:
val digitsSet = Set((10,20,30),(1,5,6),(78,109,23),(14,25,57)) println(digitsSet.contains((10,20,30)))
Output:
true
-
Adding a
return
statement beforetrue
andfalse
. This is the best way as it is versatile and can be used in almost any scenario.val digitsSet = Set((10,20,30),(1,5,6),(78,109,23),(14,25,57)) def check(): Boolean = { val store = for ((digit1,digit2,digit3) <- digitsSet) { if ((10,20,30) == (digit1,digit2,digit3)) return true } false } println(check())
Output:
true
-
Using the
exists
method in Scala.val digitsSet = Set((10,20,30),(1,5,6),(78,109,23),(14,25,57)) println(digitsSet.exists( _ == (1,20,50) ))
Output:
false