How to Log in a Scala Application
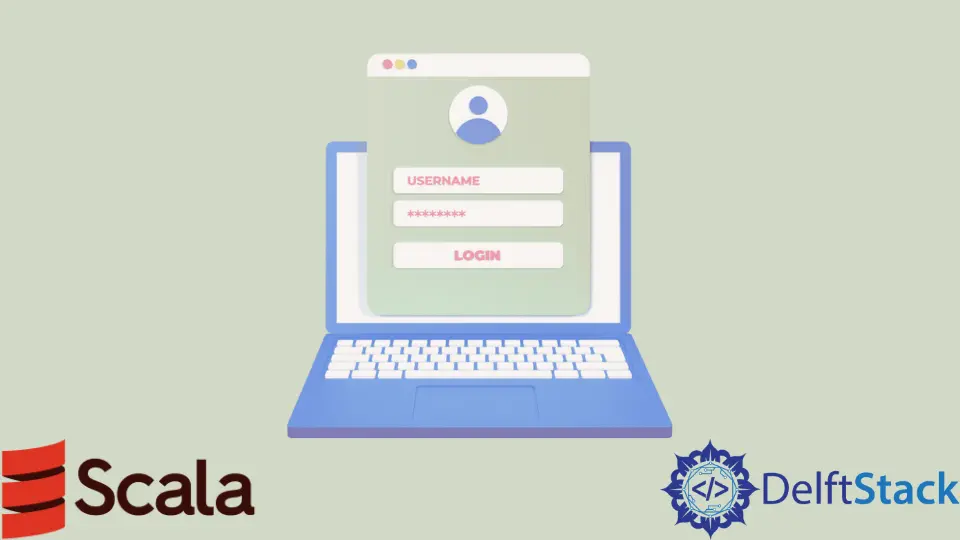
This article will introduce how to do application logging in Scala.
Use the logger
Class to Do Logging in a Scala Application
We can use the logger
class to do application logging in Scala. It is present inside the com.typesafe.scalalogging
package, and it also internally wraps the Simple Logging Facade for Java (SLF4J) logger.
We’ll see an example for logging using one of the logging frameworks, log4j
. But to include it in our application, first, we have to perform the following steps.
-
Update the
build.sbt
file present in the project file belowlog4j
dependencies.libraryDependencies += "log4j" % "log4j" % "1.2.14"
-
Create
Log4j.properties
file undersrc/main/resources
and add the following:# Define the root logger with appender file log = D:/hero #GIVE YOUR PATH WHERE YOU WANT THE LOG FILE TO BE CREATED log4j.rootLogger = DEBUG, FILE # Define the file appender log4j.appender.FILE=org.apache.log4j.FileAppender log4j.appender.FILE.File=${log}/log.out # Define the layout for file appender log4j.appender.FILE.layout=org.apache.log4j.PatternLayout log4j.appender.FILE.layout.conversionPattern=%m%n
Now, we can use it in our code below.
import org.apache.log4j.Logger
object workspace {
val logger = Logger.getLogger(this.getClass.getName)
def main(args: Array[String]): Unit = {
logger.info("We are logging in Scala")
}
}
Output:
Logger: We are logging in Scala
When the above program is executed, the log file log.out
is created in the D:/hero
specified in the Log4j.properties
folder.
Levels of Logging in Scala
In Scala, we have three levels of logging: debug
, info
, and error
. We also have the trace
, but it is just a detailed debugging version.
Info Logging in Scala
This gives information to the user about the activity or events the application has performed. Here we can put logs like application started
, database connection established
, finished execution
, etc.
Error Logging in Scala
This level tells us that there is some error or problem in our application. It could be anything like service not running
, failed to establish a database connection
, or some other kind of failure.
Debug Logging in Scala
This level helps in performing diagnosis regarding the application. Once we have found an error in the application, we have to debug
, meaning find out where or what is causing it and fix it as soon as possible.
Trace Logging in Scala
A descriptive or detailed version of the debug
level.