How to Getting List Elements in Scala
-
Accessing List Elements Using
index
in Scala -
Accessing List Elements Using the
lift()
Function in Scala -
Accessing List Elements Using a
for
Loop in Scala
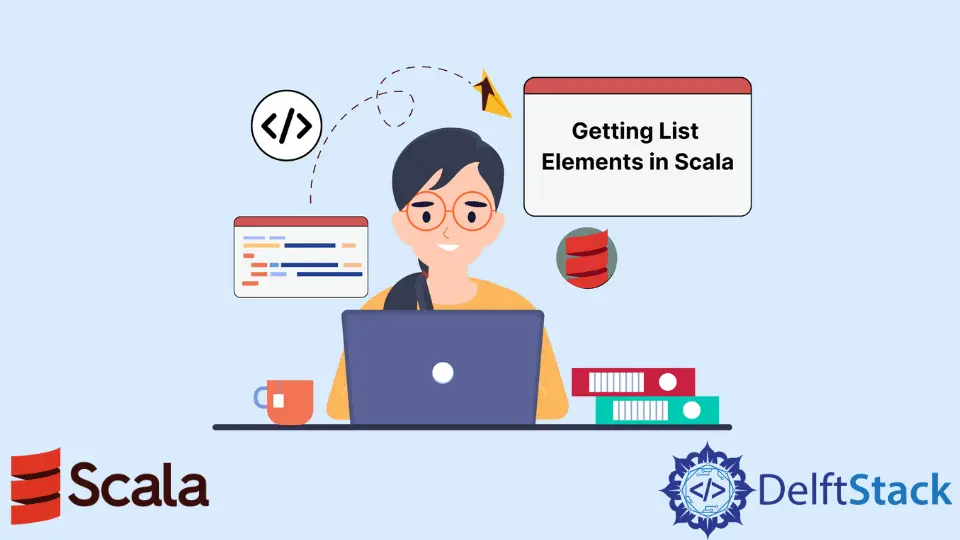
A list is a container used to store elements in linear order. In Scala, there is a class List to implement the list.
It provides several built-in functions to support its operations, such as inserting or deleting elements. This tutorial will discuss the procedure to get the list elements in Scala.
Accessing List Elements Using index
in Scala
Here, we used the index value to access the list elements. The list is an index-based data structure, so it is easy to fetch any element by passing the index only.
We access the first element using the 0
index value in the following code example.
Code:
object MyClass {
def main(args: Array[String]) {
var list1 = List("a", "b", "c")
val val1 = list1(0)
print("element: "+val1)
}
}
Output:
element: a
We can do the same for the array as well. If you have an array and want to access its elements, use the lift()
function with a valid index value.
It will return the element present in the list.
Code:
object MyClass {
def main(args: Array[String]) {
var list1 = Array("a", "b", "c")
val val1 = list1.lift(0)
println("element: "+val1)
}
}
Output:
element: Some(a)
Accessing List Elements Using the lift()
Function in Scala
The lift()
function takes integer values as an argument and returns the value present in the list. We access the 0
and 5
index values and see that since we don’t have 5 elements in the list, it returns simply none
rather than an error.
Code:
object MyClass {
def main(args: Array[String]) {
var list1 = List("a", "b", "c")
val val1 = list1.lift(0)
println("element: "+val1)
val val2 = list1.lift(5)
print("element: "+val2)
}
}
Output:
element: Some(a)
element: None
Accessing List Elements Using a for
Loop in Scala
If you want to access all the elements of the list, then use the for
loop that traverse all the list elements, and we use the print()
function to display the elements to the console screen.
Code:
object MyClass {
def main(args: Array[String]) {
var list1 = List("a", "b", "c")
for (name <- list1) println(name)
}
}