Difference Between val and var in Scala
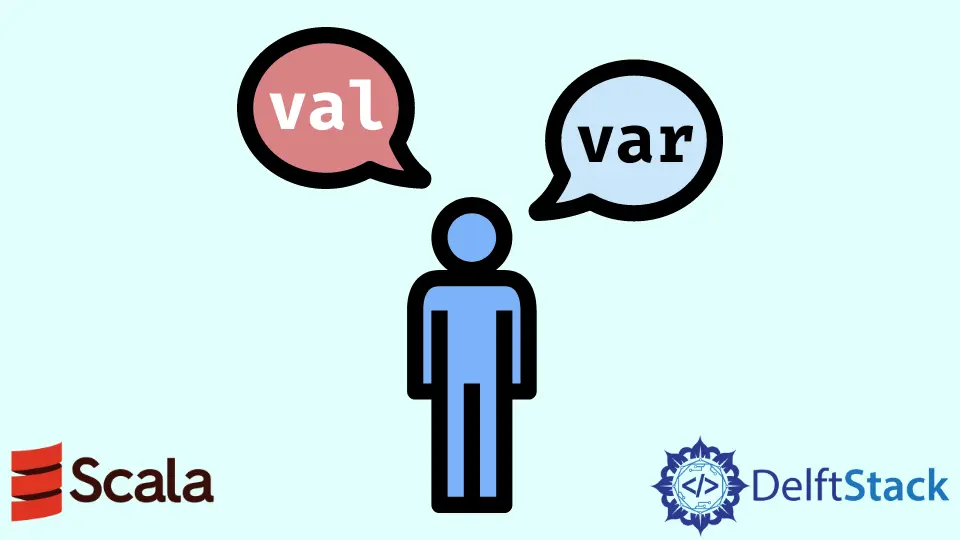
This tutorial will look at the differences between the val
and var
keywords for initializing variables in Scala.
the val
Keyword in Scala
The val
keyword is a constant reference that is immutable
, which means the value cannot be changed or modified after initialization. If we still try to change it, we will get an error, as shown below.
Code Snippet:
object MyClass {
def main(args: Array[String]) {
val name = "Iron Man"
name = "Tony Stark"
}
}
Output:
scala:7: error: reassignment to val
name = "Tony Stark"
the var
Keyword in Scala
The keyword var
is a variable that is mutable
, meaning we can change or re-assign the values to the variable. The var
keyword is useful when a variable changes constantly.
For example, suppose we have a variable account_balance
which shows the user’s current account balance. We know that this variable must be updated or changed frequently in a real-world scenario, so we must declare it as var,
not val
.
Code Snippet:
object MyClass{
def main(args: Array[String]):Unit={
var account_balance: Int = 1000
account_balance += 1000 //adding amount
account_balance -= 50 //withdrawing amount
account_balance += 200
println(account_balance)
}
}
Output:
2150
Let’s look at the differences between val
and var
keywords in a table.
val |
var |
---|---|
Values are initialized at compile time. | Values are initialized at compile time. |
Variables are mutable here. | Values are immutable here, making the variable read-only. |
Updating or changing the value does not give an error updating. | Updating or changing the value does give an error updating. |
Examples: count, loop variable, sum variable, etc. | Examples: id, social security number, etc. |