Difference Between Sequence and List in Scala
- Understanding Sequence in Scala
- Exploring List in Scala
- Key Differences Between Sequence and List
- Conclusion
- FAQ
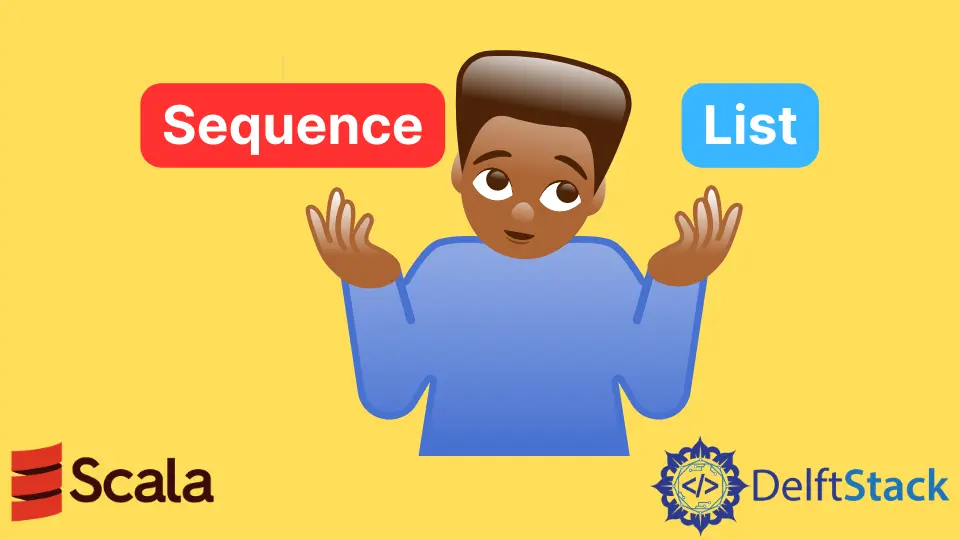
Scala is a powerful programming language that combines functional and object-oriented programming paradigms. Among its rich collection of data structures, two commonly used ones are Sequence and List. Both of these structures serve the purpose of holding collections of elements, but they have distinct characteristics and use cases.
In this article, we will explore the differences between Sequence and List in Scala, how they are utilized, and when to choose one over the other. Whether you are a beginner or an experienced Scala developer, understanding these differences is crucial for writing efficient and effective code.
Understanding Sequence in Scala
A Sequence in Scala is an abstract data type that represents an ordered collection of elements. It can contain duplicates and is indexed, allowing for easy access to its elements. Sequences can be mutable or immutable, providing flexibility depending on the requirements of your application.
The primary advantage of using a Sequence is its versatility. You can use various types of Sequences in Scala, such as Vector
, List
, and ArrayBuffer
. This means that you can choose the underlying implementation based on your performance needs. For example, if you need fast random access, a Vector
is a better choice, while if you need frequent additions and removals of elements, an ArrayBuffer
may be more suitable.
Here’s how you can create and work with a Sequence in Scala:
val seq: Seq[Int] = Seq(1, 2, 3, 4, 5)
val updatedSeq = seq :+ 6
println(updatedSeq)
Output:
List(1, 2, 3, 4, 5, 6)
In this example, we create a Sequence of integers and then add an element to it. The :+
operator appends the new element to the end of the Sequence. As you can see, working with Sequences in Scala is straightforward and allows for various operations.
Exploring List in Scala
A List in Scala is a specific type of Sequence that is immutable and represents a linked list. Lists are commonly used in functional programming due to their immutability, which helps avoid side effects and makes code easier to reason about. When you create a List, you cannot modify it; instead, you create new Lists based on existing ones.
Lists are particularly useful for recursive algorithms and when you want to maintain the original collection without altering it. They provide efficient operations for adding or removing elements from the front of the list but can be less efficient for operations that require accessing elements towards the end.
Here’s a simple example of creating and using a List in Scala:
val list: List[Int] = List(1, 2, 3, 4, 5)
val newList = list :+ 6
println(newList)
Output:
List(1, 2, 3, 4, 5, 6)
In this code snippet, we create an immutable List of integers and attempt to add an element to it. The operation creates a new List rather than modifying the original one. This behavior exemplifies the immutability characteristic of Lists in Scala.
Key Differences Between Sequence and List
Now that we have a basic understanding of both Sequence and List, let’s summarize the key differences:
- Mutability: Sequences can be mutable or immutable, while Lists are always immutable.
- Performance: Lists are optimized for operations at the front, while Sequences can be optimized for various use cases depending on their implementation.
- Flexibility: Sequences provide a broader range of data structure options, while Lists are specifically designed as linked lists.
- Use Cases: Use Sequences when you need a flexible collection that can change, and opt for Lists when you want to ensure the integrity of your data through immutability.
Understanding these differences is essential when deciding which data structure to use in your Scala applications.
Conclusion
In summary, both Sequence and List are essential data structures in Scala, each serving unique needs. Sequences offer flexibility and can be mutable or immutable, while Lists provide a functional approach with guaranteed immutability. When working with Scala, it’s crucial to choose the right data structure based on the requirements of your application. By understanding the differences between Sequence and List, you can write more efficient and effective code.
FAQ
-
What is a Sequence in Scala?
A Sequence is an ordered collection of elements in Scala that can be mutable or immutable. -
Are Lists mutable or immutable in Scala?
Lists in Scala are always immutable, meaning you cannot change them after creation. -
When should I use a List instead of a Sequence?
Use a List when you need immutability and want to avoid side effects in your code. -
Can a Sequence contain duplicate elements?
Yes, Sequences can contain duplicate elements. -
What are some common types of Sequences in Scala?
Common types of Sequences include Vector, List, and ArrayBuffer.