Difference Between :: And ::: In Scala
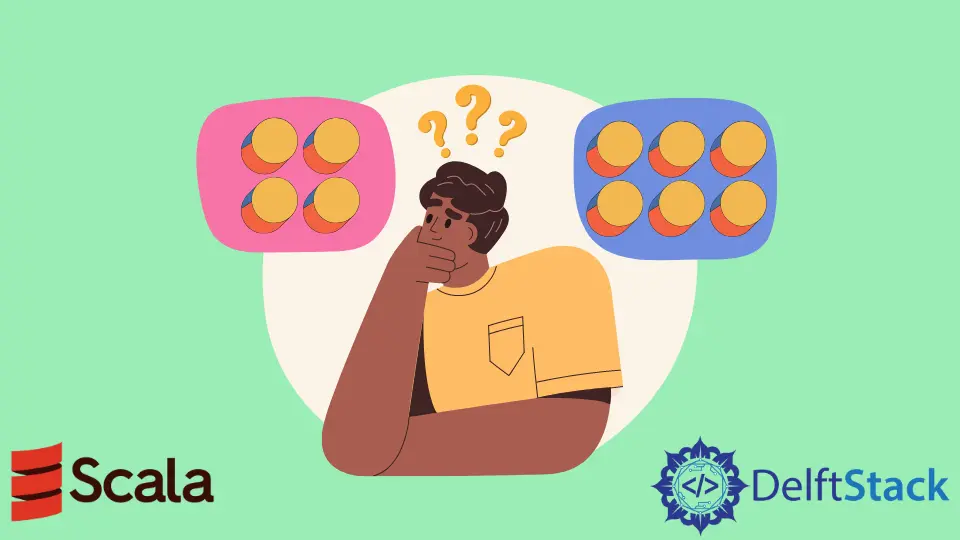
This article will differentiate between ::
and :::
in Scala, which are the operators commonly used with lists.
the ::
Operator in Scala
Scala’s ::
operator is generally used to prepend a single element to the list. It returns the list with the added element.
Definition:
def ::(x: A): List[A]
Example 1:
object MyClass {
def main(args: Array[String]):Unit= {
val fruits = List("apples", "oranges", "pears")
val basket = "Mangoes"::fruits
println(basket)
}
}
Output:
List(Mangoes, apples, oranges, pears)
Here, we can see that Mangoes
is prepended to the fruits
list.
Example 2:
object MyClass {
def main(args: Array[String]):Unit= {
val fruits = List("apples", "oranges", "pears")
val groceries = List("rice","biscuits")
val baskets = groceries :: fruits
println(baskets)
}
}
Output:
List(List(rice, biscuits), apples, oranges, pears)
We can observe that the complete groceries
list, not just its contents, are prepended to the list fruits
.
the :::
Operator in Scala
The :::
operator in Scala is used to concatenate two or more lists. Then it returns the concatenated list.
Example 1:
object MyClass {
def main(args: Array[String]):Unit= {
val fruits = List("apples", "oranges", "pears")
val groceries = List("rice","biscuits")
val baskets = groceries ::: fruits
println(baskets)
}
}
Output:
List(rice, biscuits, apples, oranges, pears)
We can observe that the list groceries
elements are concatenated to the list fruits
.
Compared to the previous example using ::
where a nested list is returned, this example using :::
returns a simple list with all the elements concatenated.
Example 2:
object MyClass {
def main(args: Array[String]):Unit= {
val fruits = List("apples", "oranges", "pears")
val groceries = List("rice","biscuits")
val flowers = List("daisy","rose","tulips")
val baskets = groceries ::: fruits ::: flowers
println(baskets)
}
}
Output:
List(rice, biscuits, apples, oranges, pears, daisy, rose, tulips)