Difference Between Object and Class in Scala
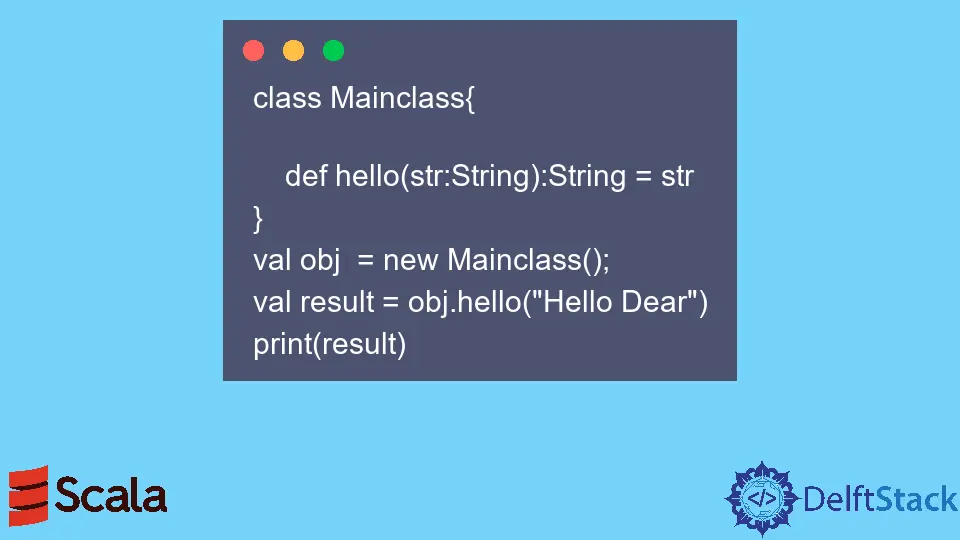
In Scala, an object is also a class with only a single instance known as a singleton object. This tutorial will discuss the difference between object and class in Scala.
Creating and Accessing Object in Scala
To create an object in Scala, we use the object keyword. An object can extend to another class To access its member fields and methods.
We can define fields and methods as we do in the case of class.
Syntax:
object <identifier> [extends <identifier>] [{ fields, methods, and classes }]
We created the MainObj
object with the hello
method. We used the object keyword to create an object and called its method without instantiating using a new keyword.
Example:
object MainObj{
def hello(str:String):String = str
}
val result = MainObj.hello("Hello Dear")
print(result)
Output:
Hello Dear
Creating and Accessing Class in Scala
Similarly, we created a class Mainclass
using the class keyword with the hello
method. We used a new keyword here to instantiate it and call its method.
Example:
class Mainclass{
def hello(str:String):String = str
}
val obj = new Mainclass();
val result = obj.hello("Hello Dear")
print(result)
Output:
Hello Dear
In conclusion, the class keyword defines a class, whereas the object keyword defines an object. A class can take parameters, whereas an object cannot.