Difference Between && and & Operators in Scala
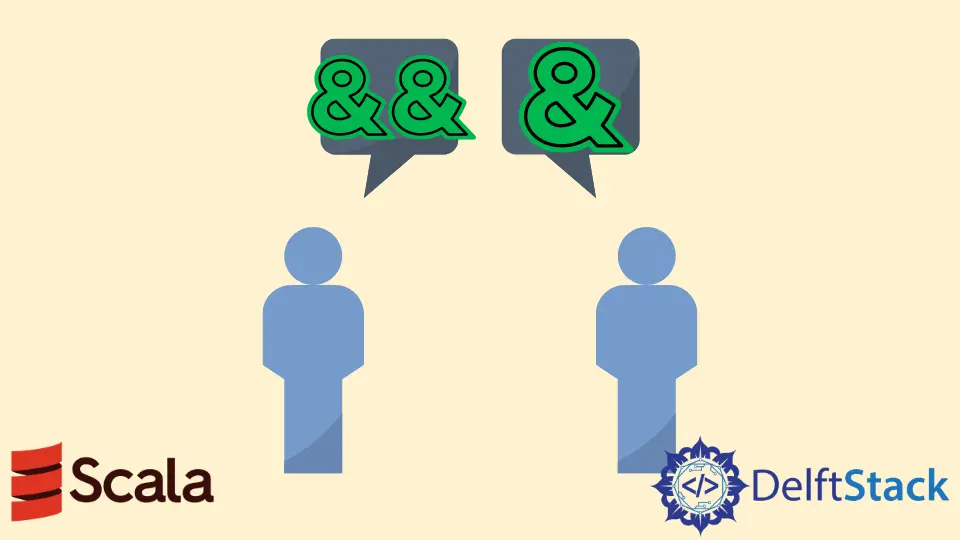
This article will discuss the difference between &&
and &
operators in Scala. The &&
and &
are both conditional operators used to test any logical expression that requires two operands and return either true
or false
, a Boolean value based on the expression result.
The &
operator is a logical and bitwise operator, as it operates on both Booleans and binary data. The &&
operator is a logical operator that works only on Boolean data type in Scala.
The &
operator evaluates both sides of the expression to get the final result. If the first expression returns false
, the &&
operator will evaluate only the left side of the expression and doesn’t evaluate the right side of the expression.
Use the Logical &&
Operator in Scala
We are comparing some integer values using the &&
operator. It returns either true
or false
.
object MyClass {
def main(args: Array[String]) {
var a = 10
var b = 20
var c = 10
if(a==b && a==c)
print("All the values are equal")
else
print("Not equal")
}
}
Output:
Not equal
Use the Bitwise &
Operator in Scala
We use the bitwise operator &
to compare some integer values. It also returns true
if both the values are true
.
object MyClass {
def main(args: Array[String]) {
var a = 10
var b = 20
var c = 10
if(a==b & a==c)
print("All the values are equal")
else
print("Not equal")
}
}
Output:
Not equal
This is the best way to check the difference between these(&&
and &
) operators. The &&
operator is also known as the short circuit operator as it does not check the second expression if the first is already false
.
Here, we increment the value of the b
variable by 1
to check whether the compiler executes the second expression or not and see the output is the same as expected.
object MyClass {
def main(args: Array[String]) {
var a=4
var b=5;
println((a == 6) && ((b=b+1) == 6));
println("b= "+b);
}
}
Output:
false
5