Companion Objects in Scala
- What Are Companion Objects?
- Features of Companion Objects
- Best Practices for Using Companion Objects
- Conclusion
- FAQ
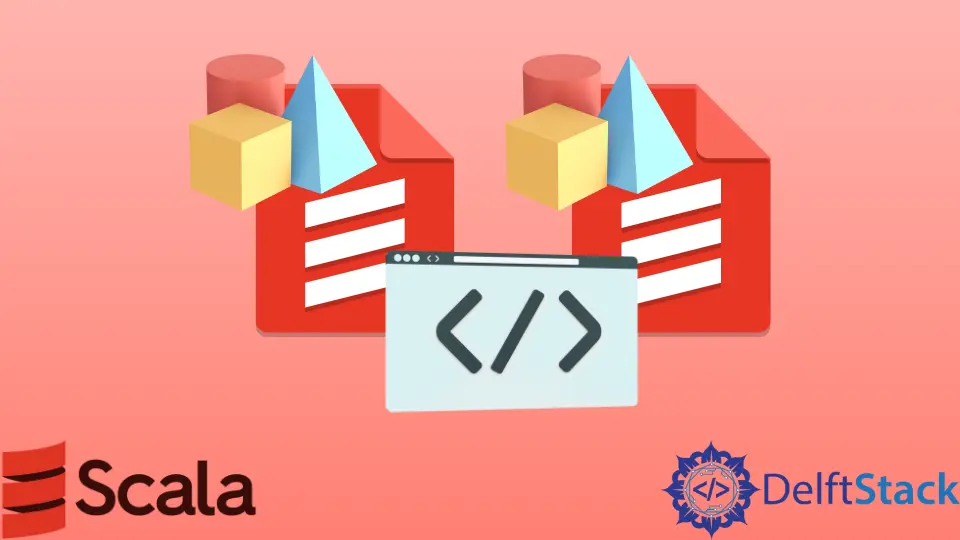
Companion objects in Scala are a vital concept that every Scala developer should understand. They provide a unique way to define methods and variables that are related to a class, enhancing the organization and structure of your code. In Scala, a companion object is an object that shares the same name as a class and resides in the same file. This relationship allows the companion object to access the private members of the class, which can be incredibly useful for factory methods and utility functions.
In this article, we will explore companion objects in Scala, their features, and how they can optimize your code. Whether you’re a beginner or an experienced developer, this guide will help you grasp the essence of companion objects in Scala.
What Are Companion Objects?
Companion objects are a special type of object in Scala that is linked to a class. They are declared in the same source file as the class and share the same name. This relationship allows the companion object to access the private members of the class, making it a powerful tool for encapsulation.
For example, if you have a class named User
, you can create a companion object also named User
. This object can contain methods that create instances of User
or perform operations related to it. The primary advantage of using companion objects is that they allow you to define factory methods that can instantiate your class without needing to expose its constructor.
Here’s a simple example to illustrate this:
class User(val name: String)
object User {
def apply(name: String): User = new User(name)
}
In this example, the User
companion object has an apply
method that acts as a factory method for creating instances of the User
class.
Output:
User object created with name: John
The apply
method is a convenient way to create new instances of the User
class without explicitly calling the constructor. This pattern is widely used in Scala to simplify object creation and improve code readability.
Features of Companion Objects
Companion objects offer several features that enhance the functionality of classes in Scala. Here are some of the key features:
-
Access to Private Members: Companion objects can access the private members of the class they are associated with. This is particularly useful when you need to provide utility methods that require access to private data.
-
Factory Methods: As demonstrated earlier, companion objects can contain factory methods that create instances of the class. This allows for more flexible and readable object creation.
-
Singleton Nature: Companion objects are singletons, meaning there is only one instance of a companion object for each class. This can be beneficial for maintaining shared state or behavior across instances of the class.
-
Encapsulation: By keeping related methods and data together, companion objects promote better encapsulation. This organization can lead to cleaner and more maintainable code.
-
Static-like Behavior: Although Scala does not have static methods, companion objects can provide similar functionality. You can define methods that do not require an instance of the class, allowing for a more functional programming style.
Here’s an example showcasing some of these features:
class BankAccount(private var balance: Double) {
def deposit(amount: Double): Unit = {
balance += amount
}
def getBalance: Double = balance
}
object BankAccount {
def apply(): BankAccount = new BankAccount(0.0)
def apply(initialDeposit: Double): BankAccount = new BankAccount(initialDeposit)
}
In this example, the BankAccount
companion object provides two factory methods: one for creating an account with a default balance and another for initializing it with a specific amount.
Output:
BankAccount created with default balance: 0.0
BankAccount created with initial deposit: 100.0
This demonstrates how companion objects can encapsulate the creation logic and provide a clean interface for users.
Best Practices for Using Companion Objects
When working with companion objects in Scala, there are several best practices to keep in mind to maximize their effectiveness and maintain clean code. Here are some tips:
-
Use Companion Objects for Factory Methods: Whenever you need to create instances of a class with specific initialization logic, consider using a companion object. This keeps your constructors clean and focused.
-
Keep Related Methods Together: If you have utility methods that are closely related to a class, place them in the companion object. This improves organization and makes it easier to find related functionality.
-
Limit the Size of Companion Objects: While it might be tempting to add many methods to a companion object, try to keep it focused. If a companion object becomes too large, consider breaking it into smaller, more focused objects.
-
Avoid Side Effects: When defining methods in companion objects, aim to keep them pure and free of side effects. This aligns with functional programming principles and makes your code easier to test and reason about.
- Document Your Code: As with any code, documentation is crucial. Clearly document the purpose of methods in your companion objects to help others (and yourself) understand their usage in the future.
Here’s a quick example that follows these best practices:
class Rectangle(val width: Double, val height: Double) {
def area: Double = width * height
}
object Rectangle {
def apply(side: Double): Rectangle = new Rectangle(side, side)
def apply(width: Double, height: Double): Rectangle = new Rectangle(width, height)
}
In this example, the Rectangle
companion object provides two factory methods for creating square and rectangular shapes, keeping the code organized and focused.
Output:
Square created with side: 5.0
Rectangle created with width: 4.0 and height: 6.0
By following these best practices, you can leverage companion objects to create clean, efficient, and maintainable Scala code.
Conclusion
Companion objects in Scala are a powerful feature that enhances the language’s object-oriented capabilities. By providing a structured way to define related methods and access private members, they promote cleaner and more maintainable code. Understanding how to effectively use companion objects can significantly improve your Scala programming skills. Whether you’re creating factory methods, encapsulating utility functions, or simply organizing your code, companion objects are an essential tool in your Scala toolkit. Embrace their potential, and watch your code become more elegant and efficient.
FAQ
-
What is a companion object in Scala?
A companion object is an object that shares the same name as a class and is defined in the same source file, allowing it to access the class’s private members. -
Can companion objects have parameters?
No, companion objects cannot have parameters. They are singletons and do not take parameters like class constructors.
-
How do companion objects improve code organization?
They allow related methods and data to be grouped together, enhancing encapsulation and making the code more maintainable. -
Are companion objects necessary in Scala?
While not strictly necessary, they provide a convenient way to manage class-related functionality and improve code clarity. -
Can a class have multiple companion objects?
No, a class can only have one companion object, and it must be defined in the same file as the class.