How to Add Elements to a Map in Scala
-
Add Elements to a Map Using the
put()
Function in Scala - Add Elements to a Map Using the Assignment Operator in Scala
-
Add Elements to a Map Using the
addOne()
Function in Scala
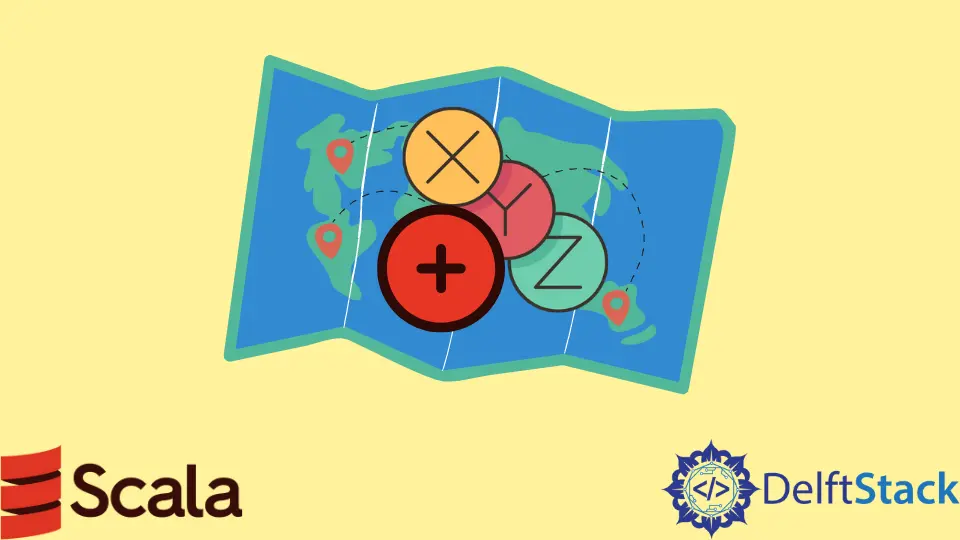
This tutorial will discuss the procedure to add an element to the Map in Scala.
The Map is a data structure used to store elements in key and value pairs. It helps manage dictionary-like information.
In Scala, to implement Map, it provides a Map class that contains several utility functions to work upon.
Let’s understand this with some running examples.
Add Elements to a Map Using the put()
Function in Scala
Here, we used the put()
function to add an element to the Map. This function takes two parameters - one is key, and the second is value.
We used for loop to check the newly added element to the Map.
Example:
object MyClass {
def main(args: Array[String]) {
val map = scala.collection.mutable.Map("k1" -> "dell", "k2" -> "mac")
for (name <- map) println(name)
println("Adding element to the map")
map.put("k3", "lenevo")
for (name <- map) println(name)
}
}
Output:
(k1,dell)
(k2,mac)
Adding element to the map
(k3,lenevo)
(k1,dell)
(k2,mac)
Add Elements to a Map Using the Assignment Operator in Scala
This is a straightforward way to add a new element to the Map. We need to use the assignment operator with the map constructor.
We need to pass the key into the constructor and assign a value using the assignment operator.
Example:
object MyClass {
def main(args: Array[String]) {
val map = scala.collection.mutable.Map("k1" -> "dell", "k2" -> "mac")
for (name <- map) println(name)
println("Adding element to the map")
map("k3") = "lenevo"
for (name <- map) println(name)
}
}
Output:
(k1,dell)
(k2,mac)
Adding element to the map
(k3,lenevo)
(k1,dell)
(k2,mac)
We can also use the +=
operator to avoid using a constructor and directly add the element to the Map.
Example:
object MyClass {
def main(args: Array[String]) {
val map = scala.collection.mutable.Map("k1" -> "dell", "k2" -> "mac")
for (name <- map) println(name)
println("Adding element to the map")
map += "k3" -> "lenevo"
for (name <- map) println(name)
}
}
Output:
(k1,dell)
(k2,mac)
Adding element to the map
(k3,lenevo)
(k1,dell)
(k2,mac)
Add Elements to a Map Using the addOne()
Function in Scala
This is another built-in function that can add elements to the Map. We can pass key and value both in the function.
Example:
object MyClass {
def main(args: Array[String]) {
val map = scala.collection.mutable.Map("k1" -> "dell", "k2" -> "mac")
for (name <- map) println(name)
println("Adding element to the map")
map.addOne("k3" -> "lenevo")
for (name <- map) println(name)
}
}