How to Split String in Rust
-
Using the
split
Method -
Using the
split_whitespace
Method -
Using the
splitn
Method -
Using the
rsplit
Method - Conclusion
- FAQ
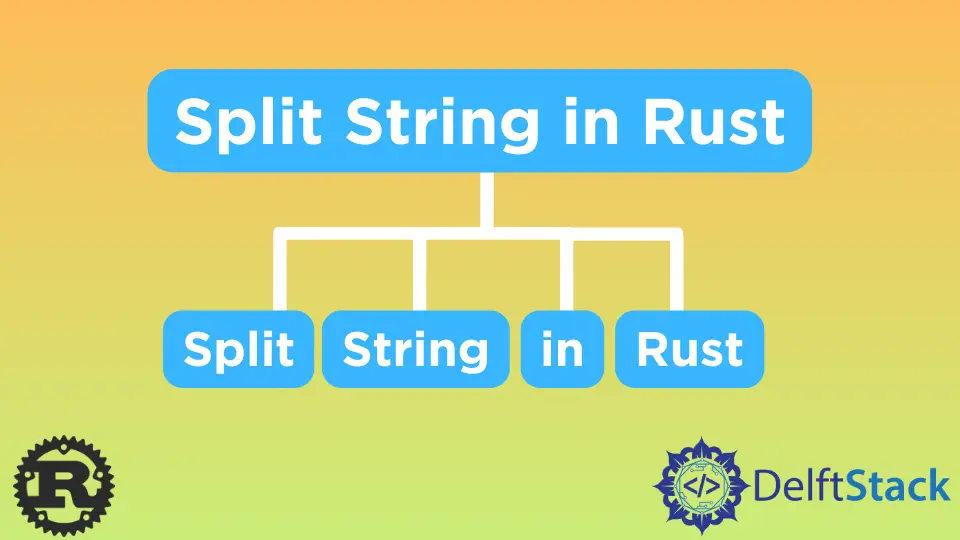
Splitting strings is a fundamental operation in programming, and Rust provides several efficient ways to achieve this. Whether you’re parsing data, processing user input, or manipulating text, understanding how to split strings in Rust can significantly enhance your coding skills.
In this tutorial, we will explore various methods for string splitting, from simple delimiters to more advanced techniques. By the end, you’ll have a solid grasp of how to handle strings in your Rust applications, making your code cleaner and more efficient. Let’s dive in!
Using the split
Method
One of the most straightforward ways to split a string in Rust is by using the split
method. This method allows you to specify a delimiter, and it will return an iterator over the substrings. Here’s a simple example:
fn main() {
let text = "hello,world,this,is,Rust";
let parts: Vec<&str> = text.split(',').collect();
for part in parts {
println!("{}", part);
}
}
Output:
hello
world
this
is
Rust
In this example, we have a string that contains several words separated by commas. By calling split(',')
, we create an iterator that divides the string at each comma. We then collect these substrings into a vector using collect()
. Finally, we loop through the vector and print each part. This method is efficient and easy to use, making it a go-to option for many developers.
Using the split_whitespace
Method
If you want to split a string based on whitespace, Rust provides the split_whitespace
method. This method automatically handles multiple spaces and returns substrings without any leading or trailing whitespace. Here’s how you can use it:
fn main() {
let text = "Rust is a systems programming language";
let parts: Vec<&str> = text.split_whitespace().collect();
for part in parts {
println!("{}", part);
}
}
Output:
Rust
is
a
systems
programming
language
In this example, the string is split into individual words based on whitespace. The split_whitespace
method is particularly useful when you’re dealing with user input or text data where spaces may vary. This method simplifies the process by ignoring empty strings and ensuring that you get only the meaningful parts of your string.
Using the splitn
Method
Sometimes, you might want to limit the number of splits performed on a string. The splitn
method allows you to specify the maximum number of splits to be made. Here’s an example:
fn main() {
let text = "one,two,three,four,five";
let parts: Vec<&str> = text.splitn(3, ',').collect();
for part in parts {
println!("{}", part);
}
}
Output:
one
two
three,four,five
In this code, we’re using splitn(3, ',')
, which will split the string at the first two commas only. The remaining part of the string is returned as a single substring. This method is particularly useful when you want to extract a fixed number of elements from a string while keeping the rest intact. It provides flexibility in string manipulation, allowing you to tailor the output to your needs.
Using the rsplit
Method
If you need to split a string but want to start from the end rather than the beginning, you can use the rsplit
method. This method works similarly to split
, but it processes the string in reverse order. Here’s how you can implement it:
fn main() {
let text = "first;second;third;fourth";
let parts: Vec<&str> = text.rsplit(';').collect();
for part in parts {
println!("{}", part);
}
}
Output:
fourth
third
second
first
In this example, we split the string using rsplit(';')
, which starts from the last semicolon and works backwards. This can be particularly useful in scenarios where the last elements of a string are more significant, such as file paths or version numbers. The rsplit
method provides a different perspective on string manipulation, allowing for more complex data extraction.
Conclusion
In this tutorial, we explored various methods for splitting strings in Rust. From the basic split
method to more advanced options like splitn
and rsplit
, Rust offers a rich set of tools for string manipulation. Understanding these methods will not only improve your coding efficiency but also enhance your ability to handle complex data. Whether you’re a beginner or an experienced developer, mastering string operations is essential for writing effective Rust applications. Happy coding!
FAQ
-
What is the difference between
split
andsplit_whitespace
?
split
divides the string based on a specified delimiter, whilesplit_whitespace
splits the string at any whitespace and ignores empty substrings. -
Can I split a string into a fixed number of parts?
Yes, you can use thesplitn
method to limit the number of splits and control how many parts you want to extract. -
How does the
rsplit
method work?
Thersplit
method splits a string from the end towards the beginning, allowing you to focus on the last elements of the string. -
Are there any performance considerations when splitting strings in Rust?
Generally, the built-in methods are efficient, but if you’re dealing with very large strings or need high performance, consider profiling your code to identify bottlenecks.
- Can I split a string using multiple delimiters?
Rust does not have a built-in method for splitting with multiple delimiters directly, but you can achieve this by using regular expressions with theregex
crate.