How to Sort a Vector in Rust
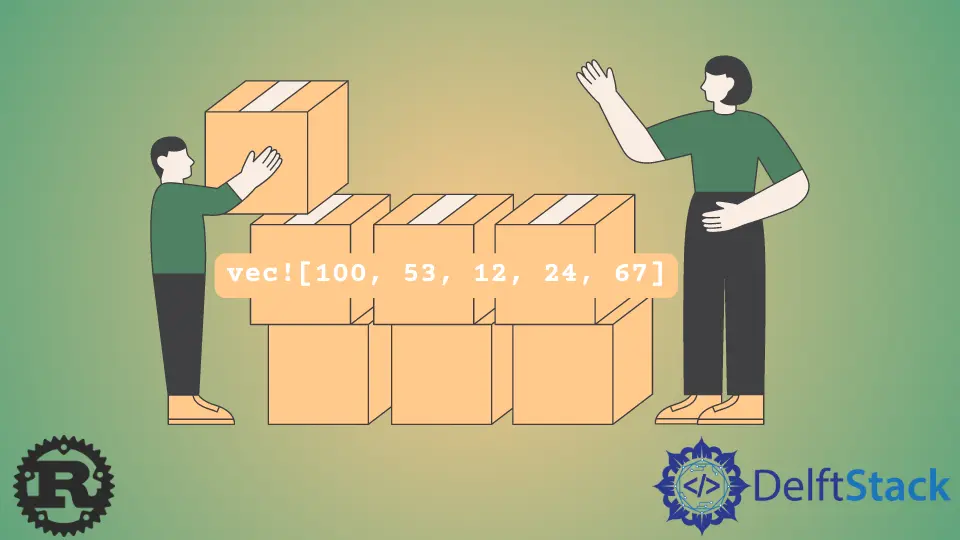
We’ll discuss the way of sorting vectors in Rust in this article.
Functions to Sort Vectors in Rust
The Rust standard library provides some valuable types for sorting vectors in Rust. There are two ways of sorting in Rust: sort_by()
function and sort()
function.
The sort_by()
function sorts the vector according to the given key, an integer or string, while the sort()
function sorts the vector according to its element’s order. The sort()
function takes about the vector and returns it sorted.
The values are sorted in ascending order by default. The sorting can be done in descending order by adding the reverse keyword before the comparison operator (<
).
Algorithm of Sorting a Vector in Rust
The sorting algorithm used by Rust is called QuickSort
. It works by dividing the vector into two halves, then recursively sorting each half until there are no more elements left to sort.
In this section, we’ll learn how to sort a vector in Rust.
- The first step is to create a vector of integers.
- The second step is to sort the elements in the vector by their values and store them in ascending order.
- The third step is to implement another function, which takes two vectors and returns an integer that represents wherein one vector would find an equal value in another vector if sorted.
- The fourth step is to call this function on every pair of elements in a given array and return a sorted array that has been sorted by index number.
We use the sort()
function to sort vector integers in the following example.
Code:
fn main() {
let mut vec = vec![100, 53, 12, 24, 67];
vec.sort();
assert_eq!(vec, vec![12, 24, 53, 67, 100]);
println!("{:?}", vec);
}
Output:
[12, 24, 53, 67, 100]
This next example uses the sort_by()
function to sort the Float vectors in Rust.
Code:
fn main() {
let mut vec = vec![1.2, 2.15, 7.5, 1.234, 5.0];
vec.sort_by(|a, b| a.partial_cmp(b).unwrap());
assert_eq!(vec, vec![1.2, 1.234, 2.15, 5.0, 7.5]);
println!("{:?}", vec);
}
Output:
[1.2, 1.234, 2.15, 5.0, 7.5]
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook