How to Implement Rust Reflection
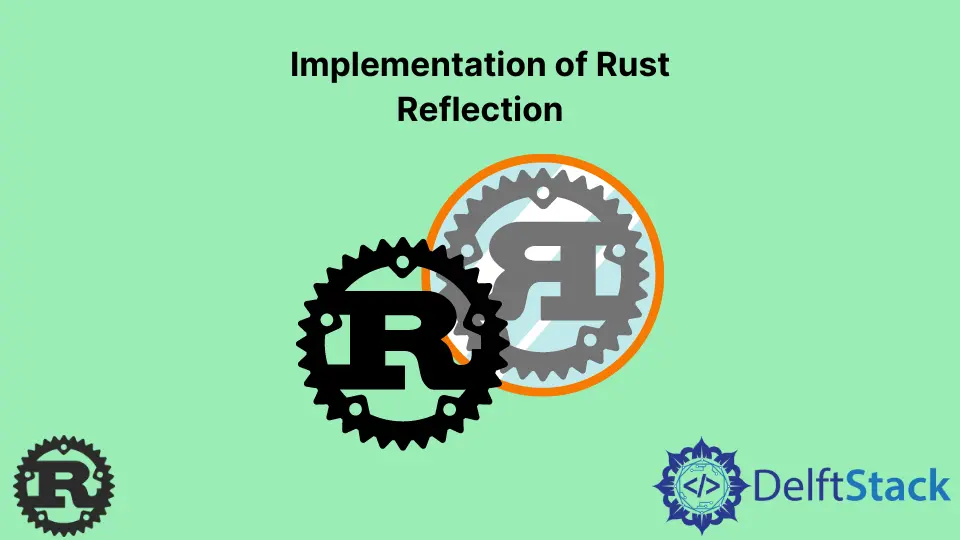
Rust is a systems programming language designed to be fast, robust and safe. Originally, Mozilla Research created it.
It has an expressive type system that guarantees memory safety without needing garbage collection and allows programmers to write programs free of data races.
Reflection
is the ability of a program to examine itself, or more, generally speaking, the ability of a program to explore other programs with which it interacts.
Rust does not have reflection built-in as part of its core functionality because it can be challenging for programmers to use correctly.
Methods to Implement Rust Reflection
One way that Rust implements reflection is through unsafe code. This may sound like an odd choice, but unsafe code can be used for many things that are usually impossible in Rust’s safe environment.
Rust also has a trait
called trait object
that is used to get details about the type at runtime. Rust’s trait
objects are similar to Java’s interface
and abstract
classes.
They allow the compiler to check at compile time that the type is correct, but they will also enable the compiler to check at runtime that the type is correct. This gives Rust a much more efficient runtime than if it had reflection.
Rust also implements generics
. Generics
create generic types, which can then be used for polymorphism.
This means that reflection in Rust uses generics
to provide the same functionality as other languages such as Python or Java have with their use of reflection.
The above mentioned are some of the methods that help implement reflection in Rust.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook