How to Make A Range in Rust
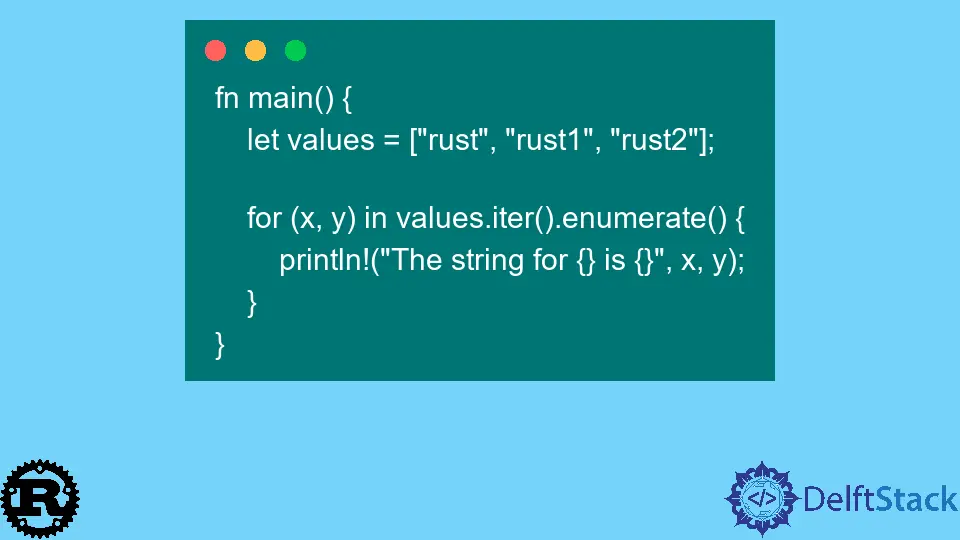
Creating a range in Rust can be a straightforward yet powerful tool for developers. Rust, known for its performance and safety features, allows you to handle ranges efficiently. Whether you’re iterating over a collection or defining limits for your data structures, understanding how to create and manipulate ranges is essential.
In this tutorial, we will explore the various methods to create a range in Rust, including syntax, examples, and practical applications. By the end of this guide, you’ll feel confident in implementing ranges in your Rust projects, enhancing your programming toolkit.
Understanding Ranges in Rust
In Rust, a range is a continuous sequence of values. It can be created using the ..
and ..=
operators. The ..
operator creates a range that excludes the upper bound, while ..=
includes it. Ranges are useful for looping through numbers, slicing arrays, and more.
Creating a Basic Range
To create a basic range in Rust, you can use the ..
operator. This operator allows you to specify a start and an end value, generating a sequence of numbers.
Here’s a simple example:
fn main() {
for i in 1..5 {
println!("{}", i);
}
}
Output:
1
2
3
4
In this example, the for
loop iterates from 1 to 4. The upper limit, 5, is excluded. This is a common pattern in Rust, especially when you want to loop through a series of numbers without including the endpoint.
Inclusive Ranges
If you need to include the upper bound in your range, you can use the ..=
operator. This is particularly useful when you need to ensure that your upper limit is part of the iteration.
Here’s how you can implement it:
fn main() {
for i in 1..=5 {
println!("{}", i);
}
}
Output:
1
2
3
4
5
In this code snippet, the for
loop iterates from 1 to 5, including both endpoints. This is ideal when you want to ensure that both the starting and ending values are part of your range.
Using Ranges with Collections
Ranges in Rust can also be used to slice collections like arrays and vectors. This allows you to access a subset of elements efficiently. Here’s an example of how to use a range to slice a vector:
fn main() {
let numbers = vec![10, 20, 30, 40, 50];
let slice = &numbers[1..4];
println!("{:?}", slice);
}
Output:
[20, 30, 40]
In this example, we create a vector of numbers and then slice it using the range 1..4
. The resulting slice contains the elements from index 1 to index 3, demonstrating how ranges can be effectively used to manipulate collections.
Ranges in Conditional Statements
Ranges can also be utilized in conditional statements, allowing for more readable code. For instance, you can check if a value falls within a specific range using the contains
method.
Here’s how you can do that:
fn main() {
let range = 1..=10;
let number = 5;
if range.contains(&number) {
println!("{} is within the range.", number);
} else {
println!("{} is out of the range.", number);
}
}
Output:
5 is within the range.
In this code, we define a range from 1 to 10 and check if the variable number
is included in that range. The contains
method provides a clean and efficient way to perform this check.
Using Step Ranges
Rust also allows you to create step ranges using the step_by
method. This is particularly useful when you want to skip certain numbers in your iteration.
Here’s an example:
fn main() {
for i in (1..10).step_by(2) {
println!("{}", i);
}
}
Output:
1
3
5
7
9
In this case, the loop iterates through the range from 1 to 9, but only prints every second number. The step_by
method enhances the flexibility of ranges, making it easier to control the flow of your loops.
Conclusion
Creating and manipulating ranges in Rust is an essential skill for any developer looking to harness the full potential of the language. Whether you’re iterating through numbers, slicing collections, or performing conditional checks, understanding how to use ranges effectively will enhance your coding efficiency. With the examples provided in this tutorial, you’re now equipped to implement ranges in your Rust projects confidently. Happy coding!
FAQ
-
What is a range in Rust?
A range in Rust represents a continuous sequence of values, defined using the..
and..=
operators. -
How do I create an inclusive range in Rust?
You can create an inclusive range by using the..=
operator, which includes the upper limit in the range. -
Can I use ranges to slice arrays in Rust?
Yes, ranges can be used to slice arrays and vectors in Rust, allowing you to access subsets of elements efficiently. -
What is the purpose of the
step_by
method?
Thestep_by
method allows you to iterate through a range while skipping a specified number of elements, providing more control over the iteration process. -
How do I check if a number is within a range in Rust?
You can use thecontains
method on a range to check if a specific number falls within that range.