How to Compile Error When Printing an Integer in Rust
- Understanding the Compile Error
- Solution: Correctly Formatting Integers
- Solution: Using the Debug Trait
- Solution: Converting Integers to Strings
- Conclusion
- FAQ
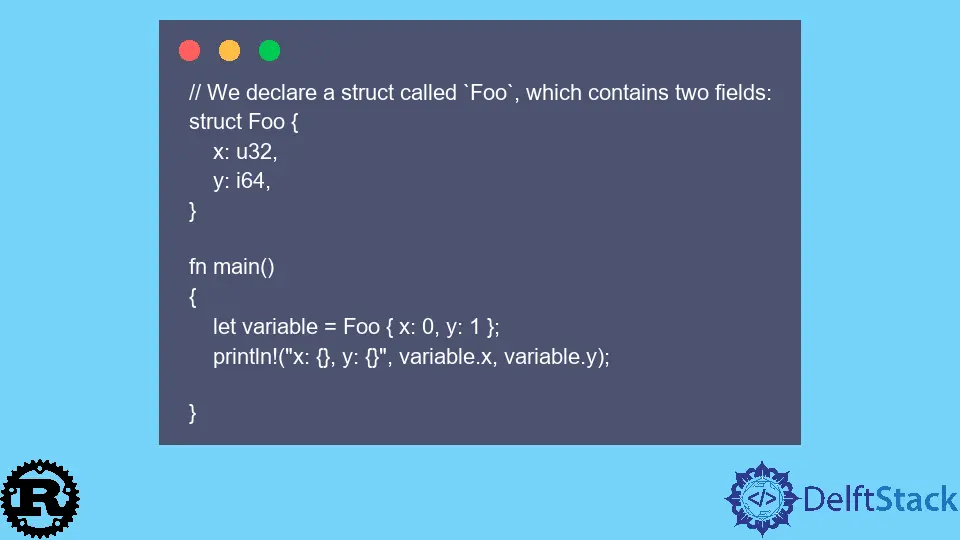
When working with Rust, especially in its earlier versions like v0.13.0, you might encounter a frustrating compile error when trying to print integers. This error typically arises from a misunderstanding of how Rust handles types and formatting. Fear not!
In this tutorial, we will walk through the common pitfalls and provide you with solutions to avoid these compile errors when printing integers in Rust. By the end of this article, you will have a solid understanding of how to correctly print integers, ensuring your Rust code runs smoothly and efficiently.
Understanding the Compile Error
Before diving into solutions, let’s clarify what the compile error looks like. When you try to print an integer using the println!
macro without proper formatting, Rust will throw an error. For example, if you attempt to print an integer directly without converting it to a string, you will encounter a type mismatch error. This is because Rust is a statically typed language, and it requires explicit type conversions in many scenarios.
Let’s see a common example that leads to this error:
fn main() {
let number = 42;
println!("The number is: {}", number);
}
Output:
The number is: 42
In this case, the code is correct, and it will compile without issues. However, if you mistakenly try to print an integer without the proper syntax, you will see a compile error.
Solution: Correctly Formatting Integers
The most straightforward way to avoid the compile error when printing integers in Rust is to ensure you are using the correct formatting syntax with the println!
macro. Rust uses curly braces {}
as placeholders within the string, and you need to provide the corresponding variables after the string.
Here’s a correct example:
fn main() {
let number = 42;
println!("The number is: {}", number);
}
Output:
The number is: 42
In this code, the println!
macro takes a format string and the variable number
as arguments. The {}
acts as a placeholder for the value of number
. Rust automatically converts the integer to a string for display, allowing for smooth compilation and execution.
The key takeaway here is to always use the correct formatting syntax. When using println!
, ensure that you include the curly braces for each variable you want to print. This approach not only prevents compile errors but also enhances code readability and maintainability.
Solution: Using the Debug Trait
Another method to avoid compile errors when printing integers in Rust is to utilize the {:?}
formatting specifier, which leverages Rust’s Debug trait. This is particularly useful when you want to print more complex data types, but it works seamlessly with integers as well.
Here’s how you can implement it:
fn main() {
let number = 42;
println!("The number is: {:?}", number);
}
Output:
The number is: 42
In this example, we use {:?}
instead of {}
. This tells Rust to use the Debug representation of the variable. While this is more commonly used for structs and enums, it works perfectly for integers too.
Using the Debug trait can be especially helpful during development. It provides a straightforward way to inspect values, making debugging easier. However, keep in mind that for production code, you may want to revert to the standard formatting with {}
for a cleaner output.
Solution: Converting Integers to Strings
If you find yourself needing to perform string manipulations before printing an integer, you can convert the integer to a string explicitly. This approach can be useful when you want to concatenate the integer with other strings or when you’re working with complex formatting requirements.
Here’s how to convert an integer to a string:
fn main() {
let number = 42;
let number_string = number.to_string();
println!("The number is: " + &number_string);
}
Output:
The number is: 42
In this code, we first convert the integer number
to a string using the to_string()
method. This method is available on all integer types in Rust. We then concatenate the string with the rest of the message using the +
operator.
This method not only helps in avoiding compile errors but also allows for more complex string operations. However, be cautious with performance in scenarios involving large numbers of conversions or concatenations, as this can impact efficiency.
Conclusion
In conclusion, encountering compile errors while trying to print integers in Rust can be frustrating, especially in earlier versions like v0.13.0. However, by understanding the correct formatting syntax, utilizing the Debug trait, and converting integers to strings when necessary, you can avoid these pitfalls. Each of these methods ensures that your Rust code is both functional and efficient. With these strategies in your toolkit, you can confidently print integers and enhance your overall Rust programming experience.
FAQ
-
What is the common compile error when printing integers in Rust?
The common error occurs when you try to print an integer without using the correct formatting syntax, leading to a type mismatch. -
How do I correctly print an integer in Rust?
Use theprintln!
macro with curly braces{}
as placeholders for the integer variable, like this:println!("The number is: {}", number);
. -
What is the Debug trait in Rust?
The Debug trait allows you to print a variable’s representation using the{:?}
formatting specifier, which can be helpful for debugging. -
Can I convert an integer to a string in Rust?
Yes, you can convert an integer to a string using theto_string()
method, which allows for string manipulations before printing. -
Are there performance concerns with string conversions in Rust?
Yes, excessive conversions and concatenations can impact performance, so it’s wise to use them judiciously in performance-critical code.