How to Use Serde to Serialize Structs Containing Ndarray Fields
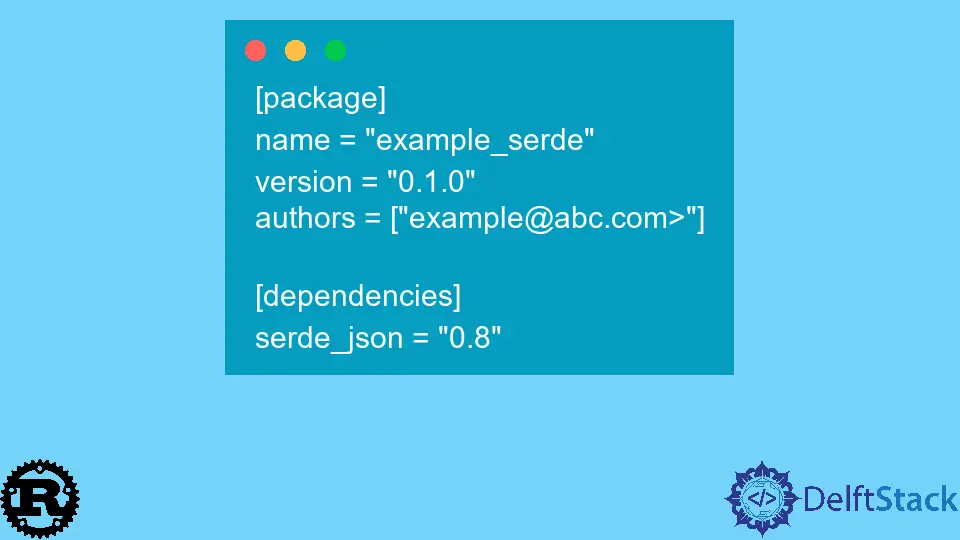
In this article, we will learn about using serde
to serialize structs containing ndarray
fields.
Create serde
Arrays in Rust
Use Serde to serialize and deserialize arrays that are either const
, generic or arbitrarily huge. Serde is a strong framework that eliminates the need for runtime type information by allowing serialization libraries to serialize Rust data structures generically.
Furthermore, in many contexts, the handshake protocol normally used between serializers and serializers can be fully optimized, allowing Serde to operate at about the same speed as a serializer hand-designed for a particular type.
Serde has support for a wide variety of types; however, it does not provide support for arrays that use const
generics. This library offers a module that, when used in conjunction with the with
attribute of Serde, adds support for the functionality.
Example of Serde in Rust
The following is a straightforward demonstration of generating and parsing JSON with the help of the serde
JSON library, which, behind the scenes, makes use of Serde. Let’s begin with the Cargo.toml
file to get things rolling.
[package]
name = "example_serde"
version = "0.1.0"
authors = ["example@abc.com>"]
[dependencies]
serde_json = "0.8"
[dependencies]
ndarray = { version = "0.13.1", features = ["serde"] }
In the previous example, the in-memory representation of the JSON value was a serde json::Value
. However, Serde can also serialize to and from standard Rust types.
Serde possesses highly effective code generation libraries compatible with Stable and Nightly Rust. These libraries remove a significant portion of the difficulty associated with manually rolling out serialization and deserialization for a specific type.