How to Match a String Against String Literals
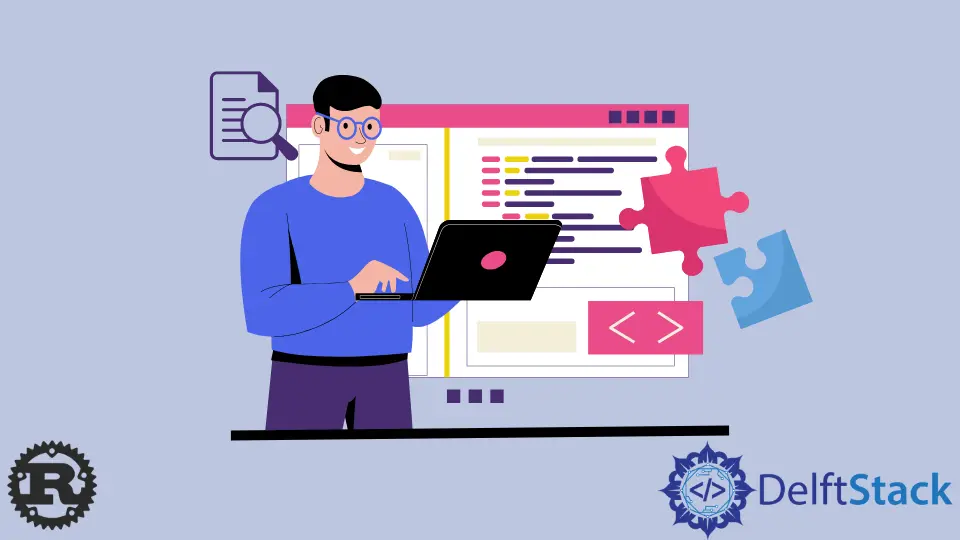
This tutorial is about matching a string against string literals in Rust.
String in Rust
The String
type is the most popular string type, as it owns the string’s contents. It is closely related to the primitive str
.
In Rust, strings are classified into two types, i.e., String
and &str
.
A String
is saved as a vector of bytes (Vec<u8>
) but is guaranteed to be a valid UTF-8 sequence. String
is a heap-allocated variable that is expandable and not null-terminated.
&str
is a slice (&[u8]
) that always points to a valid UTF-8 sequence and can be used to view into a String
, similarly to how &[T]
can be used to view into a Vec<T>
.
We can create a String
from a literal string using String::from
.
Example:
let _welcome = String::from("Welcome to the world of Rust");
String Literals in Rust
There are numerous ways to express string literals that contain special characters. They all result in a similar &str
; therefore, it’s better to use the most convenient form.
Occasionally, there are too many characters to escape, or it is more convenient to type a string out. This is the case when raw string literals are used.
Example:
fn main() {
let rawString = r"This is an example of raw string: \x3F \u{211D}";
println!("{}", rawString);
let spec_char = r#"I learnt that: "Quotes can also be used!""#;
println!("{}", spec_char);
}
Output:
This is an example of raw string: \x3F \u{211D}
I learnt that: "Quotes can also be used!"
If we need to add quotes in a raw string, we need to add a pair of #
as we have used in the above lines of code.
String literals can have line breaks in them. When a line ends, it can be a newline (U+000A)
or a pair of carriage returns and a newline (U+10000, U+10000)
.
A notable exception happens when an unescaped U+005C
character appears right before a line break. The U+005C
character and all whitespace on the following line are not translated to U+000A
.
Convert String to an &str
in Rust
We need to use .as_str()
like this for converting the String
to an &str
.
Example:
fn main() {
let the_string = String::from("z");
match the_string.as_str() {
"x" => println!("Hello"),
"y" => println!("There"),
"z" => println!("Hello Rust!!!"),
_ => println!("something else!"),
}
}
Output:
Hello Rust!!!
.as str()
is briefer and enforces more robust type checking.