Garbage Collector in Rust
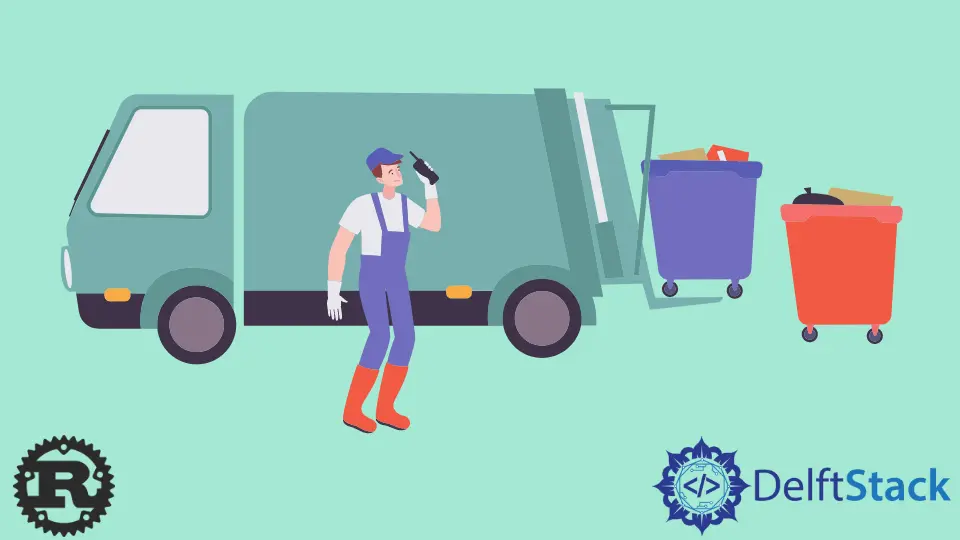
This article will teach about what Rust uses instead of a garbage collector.
Garbage Collection in Rust
Rust is a general-purpose programming language. Instead of a garbage collector, Rust achieves these properties via a sophisticated but complex type system.
This makes Rust extremely efficient but relatively difficult to learn and use.
Typically, garbage collection occurs on a periodic or as-needed basis, such as when the trash heap is nearly full or exceeds a certain threshold. Depending on the algorithm, it then searches for unused variables and releases their memory.
For example, Rust would insert the corresponding LLVM/assembly
instructions to free the memory when the variable leaves the program’s scope or its lifetime expires at compile time. Rust also supports garbage collection techniques, such as atomic reference counting.
Using an affine type system, it monitors which variable is still holding onto an object and calls its destructor when that variable’s scope expires. The affine type system can be observed in the below operation.
fn main() {
let p: String = "Good Morning".into();
let m = p;
println!("{}", p);
}
Yields:
<anon>:4:24: 4:25 error: use of moved value: `p` [E0382]
<anon>:4 println!("{}", p);
<anon>:3:13: 3:14 note: `p` moved here because it has type `collections::string::String`, which is moved by default
<anon>:3 let m = p;
^
The above yields perfectly demonstrate that ownership is tracked at all times at the language level.
Rust employs a relatively novel approach to memory management that incorporates the concept of memory ownership. Rust tracks can read and write to memory.
It detects when the program uses memory and releases it when it is no longer required. It enforces memory rules at compile time, making memory bugs at runtime virtually impossible.
There is no need to track memory manually. Instead, the compiler is responsible for it.
Rust’s most distinctive characteristic, ownership, has profound implications for the rest of the language. It is essential to understand how ownership works because it enables Rust to provide memory safety guarantees without a garbage collector.
Rust takes a different approach: memory is automatically returned when the variable that owns it exits the scope.