How to Execute Rust Diesel ORM Queries
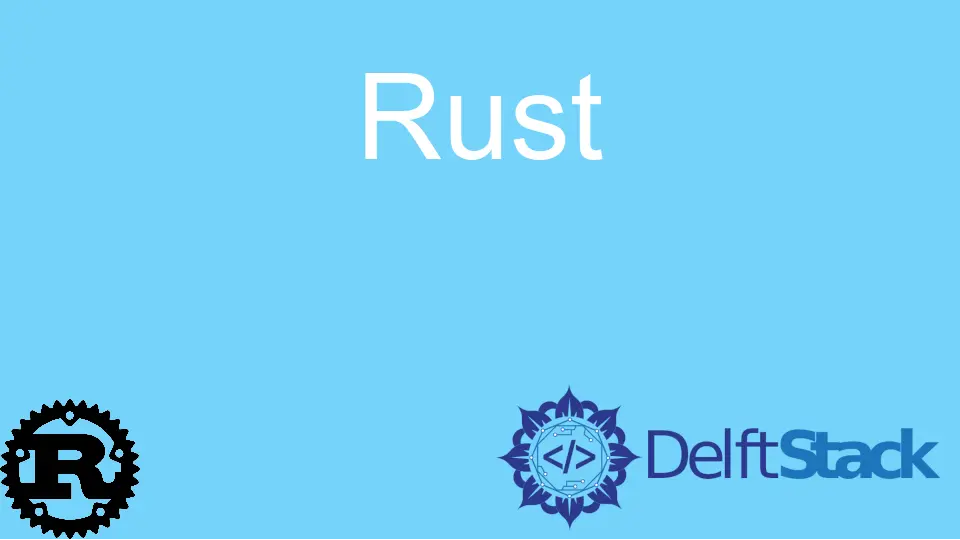
ORM assists in creating data schemas and connections within an application so that when we need to modify a specific database field, we need to alter it in our program with a few source codes. It saves time and effort in rebuilding databases every time the database structure changes.
Many languages, including Rust, JavaScript, and Ruby, implement the idea of ORM. The Diesel framework, for example, is used by Rust to assist in writing database queries within your Rust app.
Rust Diesel ORM Queries
Rust Diesel is an ORM framework for Rust that provides an object-relational mapping layer between the relational database and the rust code. The framework supports multiple databases, including MySQL, PostgreSQL, SQLite, MSSQL, and Oracle.
The object-relational mapping layer can be used for querying in Rust Diesel ORM with the help of queries like select()
, update()
, delete()
, insert()
, etc. Rust’s Diesel ORM lets you construct complex queries using Rust types and methods.
It offers the following features:
- Queries are constructed by combining primitive Rust types such as strings, integers, booleans, etc.
- Queries can be built up from these primitive types in any order.
- Queries can be parameterized with values.
- Queries can be combined with boolean operators (
AND
,OR
).
Set Up the Rust App
Rust uses Cargo, a package manager that allows us to access, install, and utilize external libraries in our applications, to install and run apps. It is installed alongside the Rust compiler.
Browse to the chosen place and run the following command to install the Rust app.
cargo new todos-graphql-api
After that, you need to follow the following steps to proceed.
Steps to Execute Rust Diesel ORM Queries
The following steps are needed to execute Rust Diesel ORM queries:
-
Add the following lines to your
Cargo.toml
file.[dependencies] diesel = "2.0"
-
Import Diesel in your
lib.rs
ormain.rs
file.use diesel::prelude::*;
-
Create a
struct
that will be used as the table’s primary key and add it to your table definition in the database.struct UserId { id: i32, }
-
Define a
struct
for each table field you want to query on, and include it in the query method call.s#users{id}#name#age#;
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook