How to Make An Interactive Debugger for Rust
- Setting Up Your Rust Environment
- Building the Interactive Debugger
- Implementing Command Handling
- Integrating with Rust Debugging Libraries
- Conclusion
- FAQ
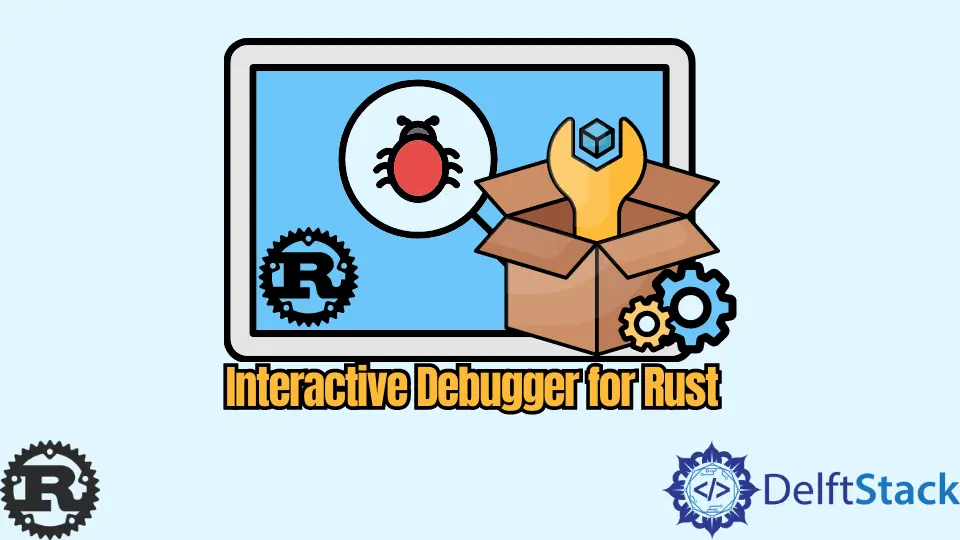
Creating an interactive debugger for Rust can significantly enhance your development workflow. With Rust’s growing popularity for systems programming, having a reliable debugging tool is essential.
This tutorial will guide you through the process of building an interactive debugger step by step, utilizing Rust’s features and libraries. By the end of this article, you’ll be equipped with the knowledge to implement your own interactive debugger, making it easier to identify and fix issues in your Rust applications. Let’s dive in!
Setting Up Your Rust Environment
Before we start building our interactive debugger, it’s crucial to have a proper Rust development environment. If you haven’t already, you’ll need to install Rust and its associated tools. You can do this using rustup
, which is the recommended way to install Rust.
To install Rust, run the following command in your terminal:
curl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh
After installation, ensure that your Rust toolchain is up to date:
rustup update
Once your environment is set up, you can create a new Rust project. Navigate to your desired directory and run:
cargo new interactive_debugger
cd interactive_debugger
This command creates a new directory called interactive_debugger
with a basic Rust project structure. You’ll find a src/main.rs
file where you can start coding your debugger.
Building the Interactive Debugger
The first step in creating our interactive debugger is to set up a simple command-line interface (CLI) that allows users to input commands. We will utilize the std::io
library for handling user input.
Here’s a basic implementation of a CLI:
use std::io::{self, Write};
fn main() {
loop {
print!("dbg> ");
io::stdout().flush().unwrap();
let mut input = String::new();
io::stdin().read_line(&mut input).unwrap();
let command = input.trim();
if command == "exit" {
break;
} else {
println!("Executing command: {}", command);
// Here we would process the command
}
}
}
This loop continuously prompts the user for input until they type “exit”. It reads the input, trims any whitespace, and prints the command back to the user. In a complete debugger, you would replace the comment with logic to process and execute the command.
Output:
dbg> help
Executing command: help
In this code, we first import the necessary modules from the standard library. The loop
construct enables continuous interaction with the user. The print!
macro outputs the command prompt, and io::stdout().flush().unwrap()
ensures that the prompt appears immediately. The user input is then read and processed.
Implementing Command Handling
Now that we have a basic CLI, the next step is to implement command handling. This will allow our debugger to respond to various commands, such as “run”, “break”, and “continue”.
Here’s how you can implement basic command handling:
fn handle_command(command: &str) {
match command {
"run" => println!("Running the program..."),
"break" => println!("Setting a breakpoint..."),
"continue" => println!("Continuing execution..."),
_ => println!("Unknown command: {}", command),
}
}
fn main() {
loop {
print!("dbg> ");
io::stdout().flush().unwrap();
let mut input = String::new();
io::stdin().read_line(&mut input).unwrap();
let command = input.trim();
if command == "exit" {
break;
} else {
handle_command(command);
}
}
}
In this code, we define a handle_command
function that takes a string slice as input. It uses a match
statement to determine which command was entered and responds accordingly. This modular approach makes it easy to expand the debugger’s functionality.
Output:
dbg> run
Running the program...
The match
statement is a powerful feature in Rust, allowing for clear and concise control flow based on the value of command
. As you expand your debugger, you can add more commands to this function.
Integrating with Rust Debugging Libraries
To make our interactive debugger more powerful, we can integrate it with existing Rust debugging libraries, such as gdb
or rust-gdb
. This integration allows us to leverage existing debugging functionalities while maintaining our interactive CLI.
To call a command in gdb
, you can use the Command
struct from the std::process
module:
use std::process::Command;
fn run_gdb(command: &str) {
let output = Command::new("gdb")
.arg("--batch")
.arg("-ex")
.arg(command)
.output()
.expect("Failed to execute gdb");
println!("GDB Output: {}", String::from_utf8_lossy(&output.stdout));
}
fn handle_command(command: &str) {
match command {
"run" => run_gdb("run"),
"break" => run_gdb("break main"),
"continue" => run_gdb("continue"),
_ => println!("Unknown command: {}", command),
}
}
In this code, the run_gdb
function executes a gdb
command and captures its output. The Command::new("gdb")
creates a new command that runs gdb
, and the arg
method adds arguments to the command. The output from gdb
is printed back to the user.
Output:
dbg> run
GDB Output: Starting program: /path/to/your/program
This integration allows your interactive debugger to utilize the powerful features of gdb
, such as setting breakpoints and inspecting variables, enhancing the debugging experience significantly.
Conclusion
Creating an interactive debugger for Rust is an exciting project that can greatly improve your programming efficiency. By setting up a command-line interface, implementing command handling, and integrating with existing debugging libraries, you can build a robust tool tailored to your needs. As you continue to develop your debugger, consider exploring additional features like variable inspection and advanced command options. Happy coding!
FAQ
-
How do I install Rust?
You can install Rust using the command provided in the installation section of this article. Just run the command in your terminal. -
What libraries can I use for debugging in Rust?
You can use libraries likegdb
orrust-gdb
to enhance your debugging capabilities. -
Can I set breakpoints in my Rust debugger?
Yes, you can implement breakpoints by integrating with debugging libraries likegdb
, which supports setting breakpoints. -
How can I add more commands to my debugger?
You can easily expand thehandle_command
function by adding more match arms for new commands you wish to implement. -
Is this debugger suitable for production use?
While this tutorial provides a foundation, additional features and testing would be necessary for production use.