const() in Rust
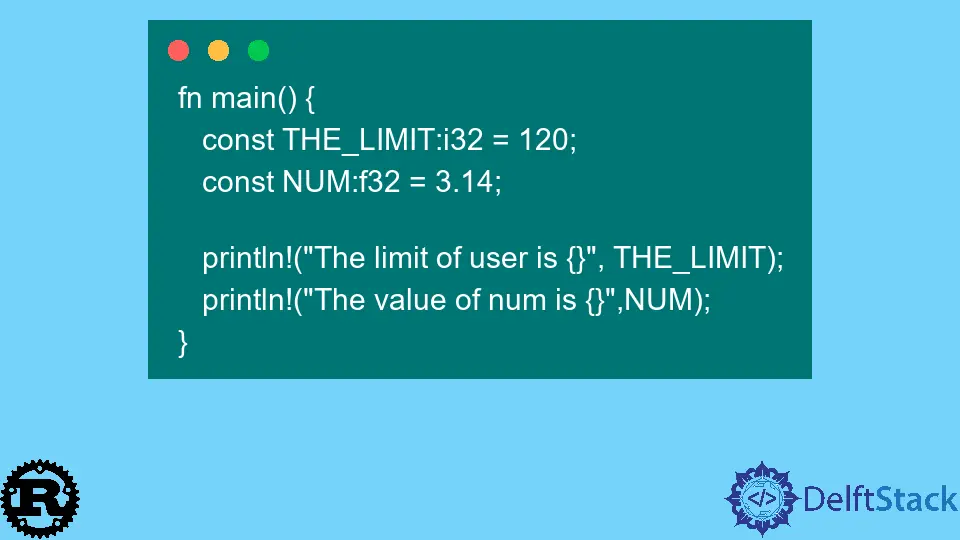
In this article, we will learn what const()
is in Rust.
const()
in Rust
When a particular value is utilized multiple times throughout a program, it can be cumbersome to copy it repeatedly. In addition, it is not always possible or desirable to make it a variable that is passed from function to function.
The const
keyword is a useful alternative to duplicating code in these cases.
Rust has two types of constants that can be declared in any scope, including global. Both require explicit annotation of type:
-
const
- an unchangeable value (the typical case) -
static
- a mutable variable with a static lifetimeThe static lifetime is deduced and is therefore not required to be specified. Accessing or altering a mutable static variable is unsafe.
Example:
fn main() {
const THE_LIMIT:i32 = 120;
const NUM:f32 = 3.14;
println!("The limit of user is {}", THE_LIMIT);
println!("The value of num is {}",NUM);
}
Output:
The limit of user is 120
The value of num is 3.14
Constants should be explicitly typed; unlike with let
, you cannot let the compiler determine their type. Any constant value could be defined in a const,
which is the vast majority of things that make sense to include in a constant; for instance, const
cannot be applied to a file.
The striking resemblance between const
and static
items creates uncertainty over when each should be used. Constants are inclined whenever used, making their usage equivalent to simply replacing the const
name with its value.
In contrast, static variables point to a single memory address shared by all accesses. This implies that, unlike constants, they cannot have destructors and operate as a single value throughout the codebase.
The const
keyword is also used without raw pointers, as seen by const T
and mut T.
Example:
static LANGUAGE: &str = "Rust";
const LIMIT: i32 = 10;
fn is_big(n: i32) -> bool {
n > LIMIT
}
fn main() {
let n = 16;
println!("{} is a programming language.", LANGUAGE);
println!("The limit is {}", LIMIT);
println!("{} is {}", n, if is_big(n) { "large" } else { "small" });
}
Output:
Rust is a programming language.
The limit is 10
16 is large