How to Concatenate Strings in Rust
-
Use the
concat!()
Macro to Concatenate Strings in Rust -
Use the
concat()
Method to Concatenate Strings in Rust - Use Owned and Borrowed Strings to Concatenate Strings in Rust
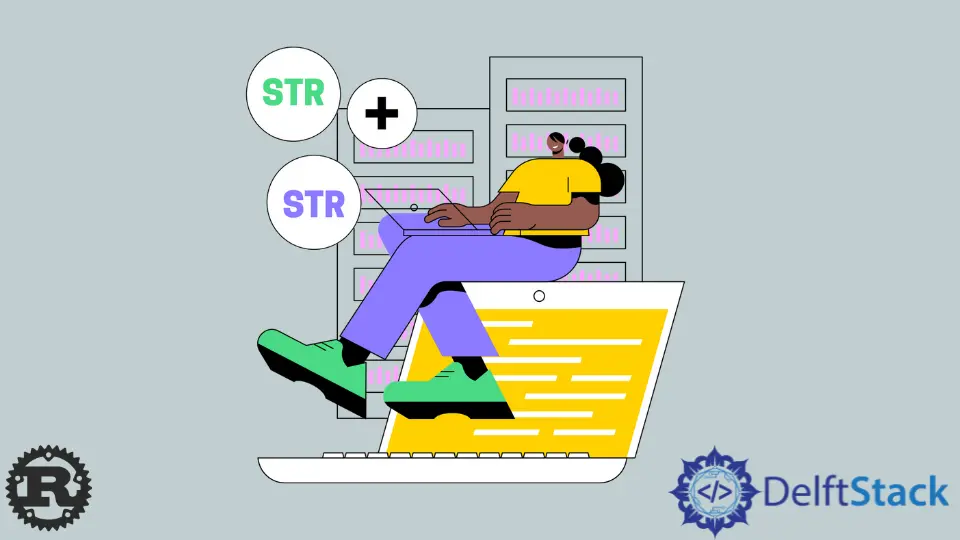
This article shows the ways to concatenate strings in Rust.
Use the concat!()
Macro to Concatenate Strings in Rust
The concat!()
is considered a macro in Rust used to concatenate two strings into a static string. Multiple comma-separated literals are needed, and the expression type for that is &'static str
.
Example Code:
fn main() {
println!("{}", concat!("bat", "man"))
}
Output:
batman
Use the concat()
Method to Concatenate Strings in Rust
Rust’s concat()
method is used to flatten a slice of type T
into a single value of type U
. You can concatenate strings with the concat()
function if it’s in a vector.
The vector will be expanded into a single string value using this approach.
Example Code:
fn main() {
let v = vec!["bat", "man"];
let s: String = v.concat();
println!("{}", s);
}
Output:
batman
Use Owned and Borrowed Strings to Concatenate Strings in Rust
We need to allocate the memory to store the result for the concatenation of the string. If the method that we are using is to modify the first string to the concatenated string, then the first string must be owned string only.
But if the strings are concatenated but not modified, then the strings can either be owned strings or borrowed strings or could be the mixture of both the strings.
To create an owned string in Rust, we need to use to_owned
as in the example code below.
let os: String = "Welcome".to_owned();
The borrowed string looks like this.
let bs: &str = "Home";
The code example for the first string is modified and then the concatenated string is:
fn main() {
let mut os: String = "Hello ".to_owned();
let bs: &str = "World";
os.push_str(bs);
println!("{}", os);
}
Output:
Hello World
Here we can mutate the string as we have an owned string. This is considered the efficient method as it allows us to reuse the memory allocation.
A similar case is available for String
and String
like &string
, which can be dereferenced as &str
.
Example Code:
fn main() {
let mut os: String = "Welcome".to_owned();
let second_os: String = "Home".to_owned();
os.push_str(&second_os);
println!("{}", os);
}
Output:
WelcomeHome
The example code used below is the one where both of the strings are borrowed.
Example Code:
fn main() {
let bs1: &str = "Welcome";
let bs2: &str = "Home";
let together = format!("{}{}", bs1, bs2);
println!("{}", together);
}
Output:
WelcomeHome