How to Get Size of an Array Passed as an Argument
- Understanding Arrays in Rust
-
Method 1: Using the
len()
Method -
Method 2: Using the
slice
Type - Method 3: Using Generics for Array Size
- Conclusion
- FAQ
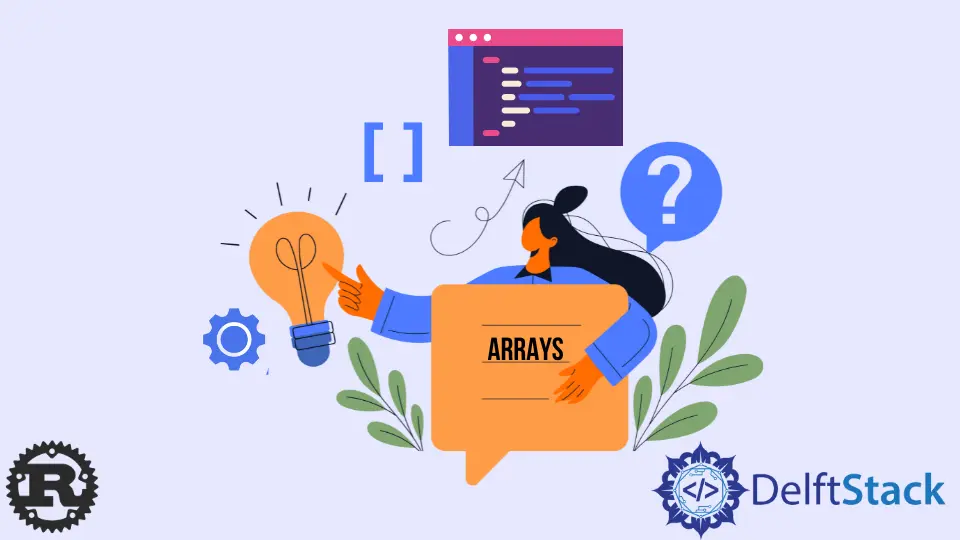
When working with arrays in Rust, one common task is determining the size of an array that is passed as an argument to a function. This can be crucial for various applications, such as ensuring data integrity and managing memory effectively.
In this tutorial, we will explore different methods to obtain the size of an array in Rust, providing clear examples and explanations along the way. Whether you are a beginner or an experienced Rustacean, understanding how to handle array sizes is a fundamental skill that will enhance your programming toolkit. Let’s dive into the world of Rust arrays and discover how to efficiently get their sizes.
Understanding Arrays in Rust
Before we jump into the methods for getting the size of an array, it’s essential to understand how arrays work in Rust. An array in Rust is a fixed-size collection of elements of the same type. The size of the array must be known at compile time, which makes arrays different from vectors that can grow or shrink dynamically. When you pass an array to a function, you often need to know its length to avoid out-of-bounds errors and to process the array correctly.
Method 1: Using the len()
Method
One of the simplest ways to get the size of an array in Rust is by using the built-in len()
method. This method returns the number of elements in the array, allowing you to easily work with its size.
Here’s an example:
fn array_size(arr: &[i32]) -> usize {
arr.len()
}
fn main() {
let my_array = [1, 2, 3, 4, 5];
let size = array_size(&my_array);
println!("The size of the array is: {}", size);
}
Output:
The size of the array is: 5
In this example, we define a function called array_size
that takes a reference to an array of integers. Inside this function, we use the len()
method to get the size of the array. When we call this function in the main
function, we pass our array, and it returns the size, which we print to the console. This method is straightforward and efficient, making it a go-to choice for many developers.
Method 2: Using the slice
Type
Another effective way to get the size of an array in Rust is to pass it as a slice. A slice is a dynamically-sized view into a contiguous sequence of elements. When you pass an array as a slice, you can still use the len()
method to get the size.
Here’s how it works:
fn array_size(arr: &[i32]) -> usize {
arr.len()
}
fn main() {
let my_array = [10, 20, 30, 40, 50, 60];
let size = array_size(&my_array);
println!("The size of the array is: {}", size);
}
Output:
The size of the array is: 6
In this example, we again define the array_size
function, which takes a slice of integers. By passing the array as a slice using the &
operator, we can still access the len()
method to get the size. This approach is particularly useful when you want to work with parts of an array without needing to know its exact size beforehand. Using slices provides flexibility while maintaining the safety and performance characteristics of Rust.
Method 3: Using Generics for Array Size
If you want to get even more advanced, you can use generics to create a function that accepts arrays of any size. This method takes advantage of Rust’s type system and allows you to define a function that can operate on arrays of different lengths.
Here’s an example:
fn array_size<const N: usize>(arr: &[i32; N]) -> usize {
N
}
fn main() {
let my_array = [1, 2, 3, 4, 5, 6, 7];
let size = array_size(&my_array);
println!("The size of the array is: {}", size);
}
Output:
The size of the array is: 7
In this example, we define the array_size
function using a const generic parameter N
, which represents the size of the array. By passing an array of a specific size, the function can return the size directly without needing to call len()
. This method is particularly useful for situations where you want to enforce compile-time checks on the size of the array, ensuring that your function can only accept arrays of the specified size.
Conclusion
Getting the size of an array passed as an argument in Rust is a fundamental skill that can help you write safer and more efficient code. Whether you choose to use the len()
method, work with slices, or leverage generics, Rust provides various approaches to suit your needs. By mastering these methods, you can enhance your programming capabilities and ensure that your applications handle data effectively. Keep experimenting with these techniques, and you’ll find that managing arrays in Rust becomes second nature.
FAQ
-
How do I get the size of a dynamic array in Rust?
You can use vectors, which are dynamic arrays, and call thelen()
method on them to get their size. -
Can I get the size of a multidimensional array in Rust?
Yes, you can get the size of each dimension separately by using thelen()
method on each array or slice. -
What happens if I try to access an index out of bounds in Rust?
Rust will panic at runtime, ensuring that your program does not run into undefined behavior. -
Is there a way to create an array with a variable size in Rust?
No, in Rust, arrays have a fixed size, but you can use vectors if you need a dynamically sized collection. -
How does Rust ensure memory safety when working with arrays?
Rust uses ownership and borrowing rules to ensure that memory is managed safely, preventing issues like dangling pointers and buffer overflows.