How to Use the Ampersand in Rust
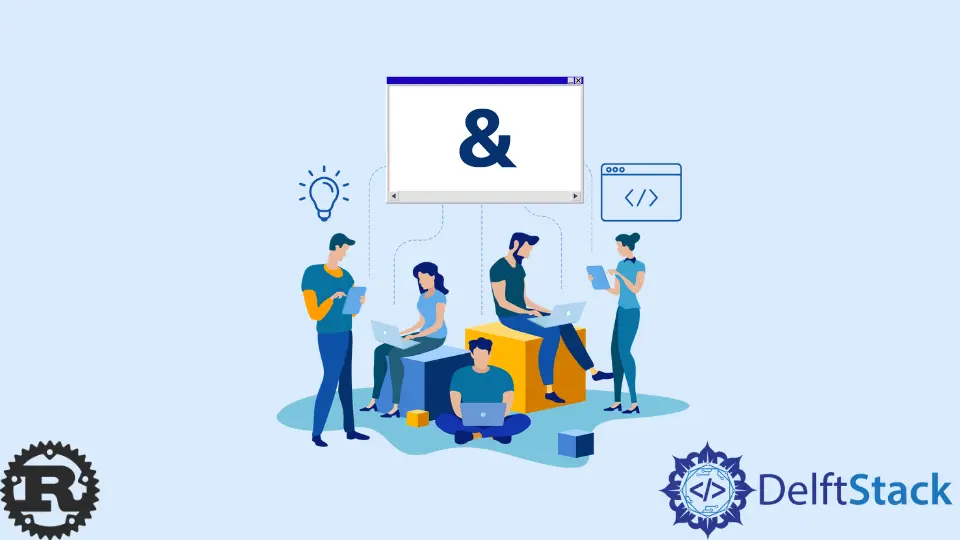
Rust is a systems programming language that emphasizes safety and speed. The compiler catches common mistakes in the code, meaning there is less debugging time and fewer security problems.
In addition to these features, Rust has an ampersand (&
) feature that can make any variable mutable or immutable at any point in the code.
Use the Ampersand (&
) to Make Mutable or Immutable Variable in Rust
When you employ the ampersand (&
) sign, it converts to a reference. The crucial idea here is that if we send a reference variable as an argument to a function, that function will take a reference argument.
You’ll notice a compile-time error if you don’t use an ampersand (&
).
Concept of Mutability
In Rust, the ampersand (&
) symbol denotes an immutable reference, and the mutable ampersand (&mut)
indicates a mutable reference.
The concept of mutability in Rust is very important to understand when using references. Many of Rust’s safety guarantees are based on this distinction between mutable and immutable references.
The mutable ampersand can be mutated and reassigned without the need for using pointers or references. This provides a more efficient way to handle mutation because it eliminates the need for many explicit copies and moves.
Let’s discuss an example.
fn main() {
let mut x = 6;
{
let y = &mut x;
*y -=2;
}
println!("A number is {}", x);
}
Output:
A number is 4
If we didn’t apply mut
in the above example, the following line would fail to execute. And if we didn’t use the mut
keyword to construct a variable at the start, the program will give an exception.
We were attempting to obtain a changeable reference from an immutable variable.
There is also an essential factor to consider: you can only have one changeable reference. As a result, you cannot construct numerous mutable references.
Click here to check the live demonstration of the code mentioned above.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook