How to Initialize Variables in a Rust Struct
-
Use
struct
to Declare and Initialize Structures in Rust -
Implement
Default
Trait in Rust -
Use
derivative
Crate in Rust
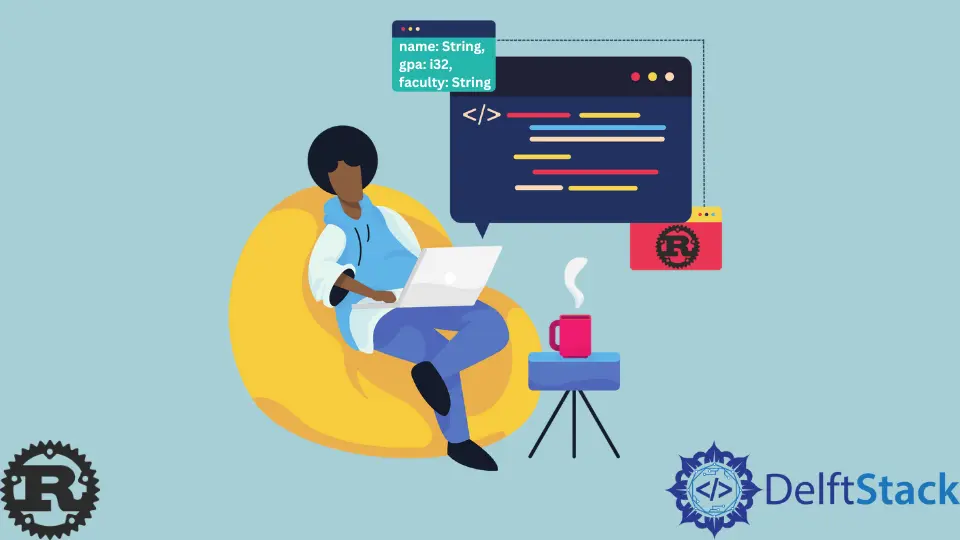
A struct
is a user-defined data type containing the fields that define the particular instance. struct
helps implement abstract ideas more understandably.
Use struct
to Declare and Initialize Structures in Rust
struct Employee {
id: i32,
name: String,
address: String,
designation: String,
}
Each field’s type is written in front of its name (e.g., id
is of type i32
, the name
is a string
, address
is also a string
, and so on). When the struct
is declared, the value should be assigned to each field known as initialization.
struct Student {
name:String,
gpa:i32,
faculty:String,
}
fn main() {
let std1 = Student {
faculty:String::from("Computer Science"),
name:String::from("Nil"),
gpa:3
};
println!("Name is :{}, Faculty is :{}, GPA is :{}",std1.name,std1.faculty,std1.gpa);
}
We created a struct
named Student
containing three fields name
, gpa
, and faculty
. The data types of fields are String
, i32
and String
, respectively.
The structure is initialized by main()
. It utilizes the println!
macro to print the values of the structure’s fields.
Output:
Name is :Nil, Faculty is :Computer Science, GPA is :3
Implement Default
Trait in Rust
Implementing the Default
trait can supply default values for the struct
. We may use an automatically produced default implementation by making a modest adjustment to the data structure.
When #[derive(Default)]
is used in a data structure, the compiler constructs a default data structure with the default value in every field. The default Boolean value is false
, while the default integral value is 0
as of Boolean.
#[derive(Default)]
struct Student {
name:String,
gpa:i32,
faculty:String,
}
fn main() {
let std1 = Student {
faculty: String::from("Computer Science"),
name:String::from("Nil"),
..Default::default()
};
println!("Name is :{}, Faculty is :{}, GPA is :{}",std1.name,std1.faculty,std1.gpa);
}
We have used a default value for gpa
. It will have a default integral value which is 0
.
Output:
Name is :Nil, Faculty is :Computer Science, GPA is :0
Use derivative
Crate in Rust
The derivative
crate adds a set of #[derive]
properties to Rust that may be customized. It uses attributes to generate more implementations than the built-in derive(Trait)
.
use derivative::Derivative;
#[derive(Derivative)]
#[derivative(Debug, Default)]
struct Student {
#[derivative(Default(value = "-1"))]
gpa:i32,
}
fn main() {
println!("Student: {:?}", Student::default());
}
We set the default value for gpa
as -1
. We will run the above code without passing any value to gpa
.
Output:
Student: Student { gpa: -1 }