How to Create Enums in Rust
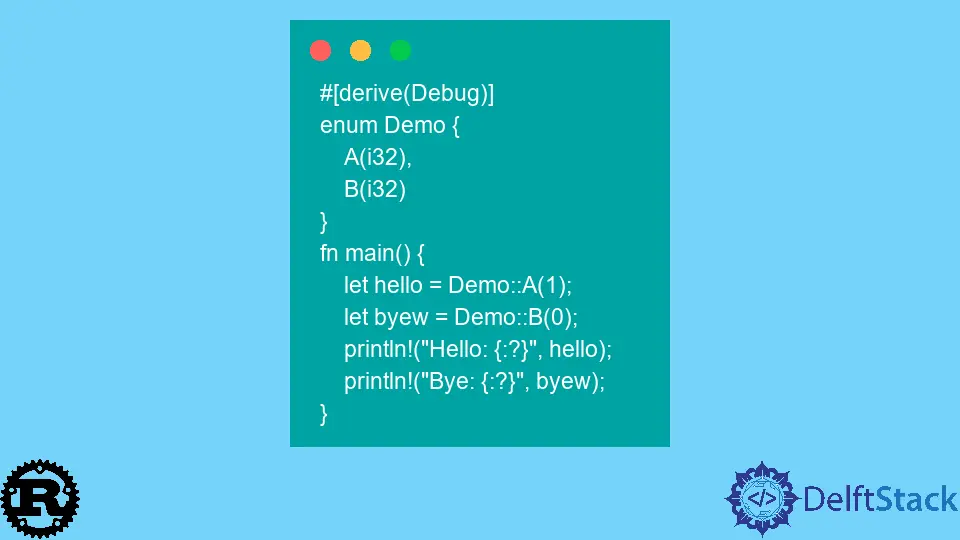
Enums
are a way of representing a set of related values. In Rust, they are implemented as an integral type with variants.
The enum
keyword is used to create an enum
type, and the variant keyword is used to declare variants. The variant can contain any number of constants called enumerators, and variants can also have methods, which are called associated functions or associated constants.
In this article, we’ll discuss the Rust Enums
concept.
Declare an enum
in Rust
The enum
keyword is used to create an enumeration. It can be used to define a type with several different values.
- Create the
enum
definition with theenum
keyword, followed by the name of the enumerated type, followed by a list of values in curly braces({}
). - Create instances of this type with the same name as the type and then assign each instance a value from its corresponding list.
- The
case
keyword is used to create branches in anenum
definition.
Initialize enum
With Values in Rust
We assign an enum
with a value to a variable to initialize it with values. The enum
name is written first, followed by the double colon operators and the enum
value name.
Finally, a value is specified within parentheses.
Example Code:
#[derive(Debug)]
enum Demo {
A(i32),
B(i32)
}
fn main() {
let hello = Demo::A(1);
let byew = Demo::B(0);
println!("Hello: {:?}", hello);
println!("Bye: {:?}", byew);
}
Output:
Hello: A(1)
Bye: B(0)
Benefits of enum
in Rust
An enum
is a data type containing a set of related values. It is like an array, but you don’t have to worry about running out of space or re-allocating memory when adding more items.
Enums
are better because they are more explicit and less error-prone than using a string for every possible value. Enums
also allow for compile-time checks that ensure you do not assign the wrong type to a variable or misspell one of the variants in your code.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook