How to Convert String to Int in Rust
Nilesh Katuwal
Feb 02, 2024
Rust
Rust Convert
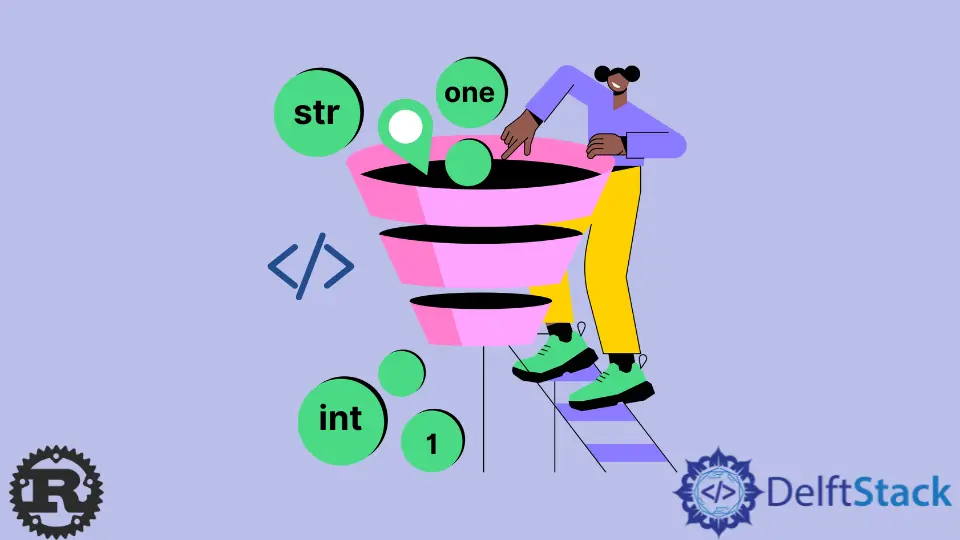
This article will teach us how to convert the string to int in rust.
Use the str::parse::()
Method to Convert String to Int in Rust
We can directly convert the string into an int using the str::parse::()
method.
let string_value = "27".to_string();
let int_value = string_value.parse::<i32>().unwrap();
Example Code:
fn main() {
let mut string_one: &str = "789";
let mut i = match string_one.parse::<i32>() {
Ok(i) => i,
Err(_e) => -1,
};
println!("{:?}", i);
}
Output:
789
Now, we will extract the integer value i
from the a
, which is a string representation. Let’s look at the following code.
Example Code:
fn main() {
let mut a = "789";
let mut i: i32 = a.parse().unwrap_or(0);
println!("{:?}", i);
a = "12u3";
i = a.parse().unwrap_or(0);
println!("{:?}", i);
}
Output:
789
0
If we want to parse to a specific type like usize
, we can use the special Rust turbofish
opertor in an if-let
statement for parsing to a usize
.
Example Code:
fn main() {
let data = "60606";
if let Ok(result) = data.parse::<usize>() {
println!("USIZE RESULT: {}", result);
}
}
Output:
USIZE RESULT: 60606
If we have some digits inside another string, we can extract a slice from that string by using the get()
function. Then, the number can be parsed.
Example Code:
fn main() {
let value = "a 6789 b";
let inner_part = value.get(2..value.len() - 2).unwrap();
println!("INNER PART: {}", inner_part);
// Tp parse inner part.
let result: u32 = inner_part.parse().unwrap();
println!("PARSED: {0}", result);
}
Output:
INNER PART: 6789
PARSED: 6789
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe