How to Write to a File in Ruby
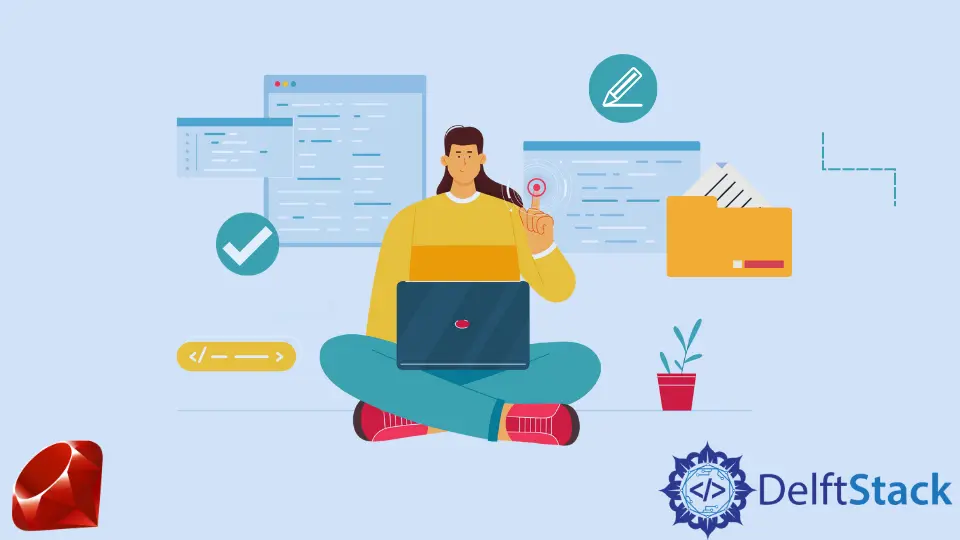
The File
class in Ruby has a write()
method for writing to a file. The method returns the length written and ensures the file is automatically closed. It has the syntax below.
File.write('/your/file/path', 'Message content')
Let’s write a simple message to a file.
File.write('my-file.txt', 'A simlpe message.')
The above code helps us create a file called my-file.txt
if it does not already exist and allows us to write A simple message.
to the file. If the file already exists, the code overwrites the file and its content.
Instead of overwriting the file content, Ruby provides a way of appending to the content by specifying the mode
option, and an example is shown below.
File.write('my-file.txt', " Another simple message\n", mode: 'a')
The \n
in the example above is a new line character, and it means the following message we’ll be writing to this file should go to the following line. Let’s write another message to confirm this.
File.write('my-file.txt', 'This message should go on the next line', mode: 'a')
As a way of summarising the explanation above, let’s write a simple program that writes five lines of text to a file.
todos = [
'wake up, shower, and leave for work',
'Pick up John from school',
'Meet with team to practice presentation',
'Dinner with friends',
'Relax and bedtime.'
]
todos.each_with_index do |todo, index|
File.write('todo-list.txt', "#{index + 1}. #{todo}\n", mode: 'a')
end
Below is the todo-list.txt
file content after running the program.
Output:
1. wake up, shower, and leave for work
2. Pick up John from school
3. Meet with team to practice presentation
4. Dinner with friends
5. Relax and bedtime.