How to Write One Line if Statement in Ruby
- Write a One-Line Statement Using the Ternary Operator in Ruby
-
Write a One-Line Statement Using
IF
Only in Ruby -
Write a One-Line Statement Using
if-else
in Ruby -
Write a One-Line Statement Using
unless
in Ruby -
Write a One-Line Statement Using
unless-else
in Ruby -
Write a One-Line Statement Using
if-else
withthen
-
Write a One-Line Statement Using
if-else
with Parentheses -
Write a One-Line Statement Using
case
for Multiple Conditions
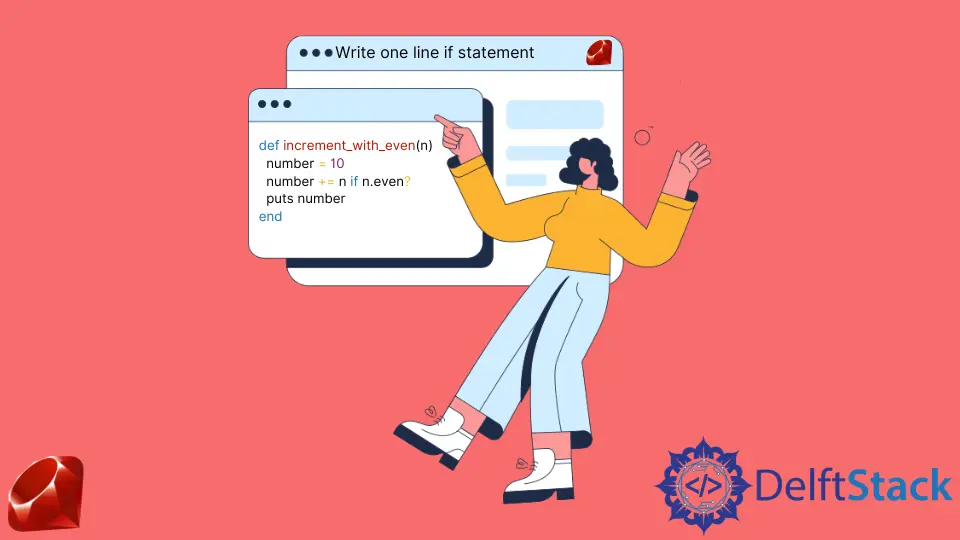
Ruby, known for its elegant and concise syntax, provides multiple ways to write one-line if
statements. In this article, we’ll discuss six methods for writing one-line if
statements in Ruby.
Write a One-Line Statement Using the Ternary Operator in Ruby
The usage of the ternary
operator is similar to how it’s used in other programming languages.
Syntax:
condition ? do_something_if_condition_is_true : do_another_thing_if_condition_is_false
Example Code:
# Define a function named 'check_even' that takes a number as an argument.
def check_even(number)
# Use a ternary operator to determine if the number is even or not, and store the result in 'response'.
response = number.even? ? "Yeah, it's even" : "No, it's not"
# Output the 'response' to the console.
puts response
end
# Call the 'check_even' function to check if it's even.
check_even(4)
check_even(5)
Output:
Yeah, it's even
No, it's not
Write a One-Line Statement Using IF
Only in Ruby
This is useful when you want to do something only if a condition is true
and do nothing if the condition is false
.
Example Code:
# Define a function named 'increment_with_even' that takes an argument 'n'.
def increment_with_even(n)
# Initialize a variable 'number' with the value 10.
number = 10
# If 'n' is even, increment 'number' by 'n'.
number += n if n.even?
# Output the value of 'number' to the console.
puts number
end
# Call the 'increment_with_even' function with the arguments 4 and 5.
increment_with_even(4)
increment_with_even(5)
Output:
14
10
Write a One-Line Statement Using if-else
in Ruby
This is a regular if-else
where you specify an action if a condition is true
and another action if it’s false
.
Example Code:
# Define a function named 'check_even' that takes a 'number' as an argument.
def check_even(number)
# Use a conditional statement to assign 'response' based on whether 'number' is even or not.
response = number.even? ? "Yeah, it's even" : "No, it's not"
# Output the 'response' to the console.
puts response
end
# Call the 'check_even' function with the arguments 4 and 5 to check if it's even.
check_even(4)
check_even(5)
Output:
Yeah, it's even
No, it's not
Write a One-Line Statement Using unless
in Ruby
The unless
is the opposite form of if
. It means if not.
# Define a function named 'increment_with_even' that takes an argument 'n'.
def increment_with_even(n)
# Initialize a variable 'number' with the value 10.
number = 10
# Increment 'number' by 'n' if 'n' is even (unless 'n' is not even).
number += n if n.even?
# Output the value of 'number' to the console.
puts number
end
# Call the 'increment_with_even' function with the arguments 4 and 5.
increment_with_even(4)
increment_with_even(5)
Output:
14
10
The usage of unless
in the above example is not recommended. Use this way for illustration.
The unless
is seen as a negative statement. In this case, it should not be used on a negative expression !n.even?
, making code more difficult to understand.
Write a One-Line Statement Using unless-else
in Ruby
def check_even(number)
response = number.even? ? "Yeah, it's even" : "No, it's not"
puts response
end
check_even(4)
check_even(5)
Output:
Yeah, it's even
No, it's not
Write a One-Line Statement Using if-else
with then
You can also use the then
keyword to create a one-line if-else
statement.
Syntax:
condition ? true_expression : false_expression
Sample Code:
grade = 85
grade >= 70 ? (puts 'Pass') : 'Fail'
Output:
Pass
In this code, the condition grade >= 70
is checked, and if it’s true, the true expression "Pass"
is executed; otherwise, the false expression "Fail"
is executed.
Write a One-Line Statement Using if-else
with Parentheses
You can wrap the expressions in parentheses for clarity in a one-line if-else
statement.
Syntax:
condition ? true_expression : false_expression
Sample Code:
x = 10
puts x > 5 ? 'x is greater than 5' : 'x is not greater than 5'
Output:
x is greater than 5
This code checks if x
is greater than 5
and prints the corresponding message accordingly.
Write a One-Line Statement Using case
for Multiple Conditions
You can use the case
statement for concise one-liners when you have multiple conditions to check.
Syntax:
result = if condition1
expression1
elsif condition2
expression2
else
default_expression
end
Sample Code:
number = 7
result = if number < 0
'Negative'
elsif number == 0
'Zero'
else
'Positive'
end
puts result
Output:
Positive
In this example, the number
is checked against multiple conditions, and the corresponding result is stored in the result
variable.