How to Use the inject Method in Ruby
- Syntax of the Inject Method in Ruby
- Sum an Array Using the Inject Method in Ruby
- Build a Hash from an Array Using the Inject Method in Ruby
- Filter an Array Using the Inject Method in Ruby
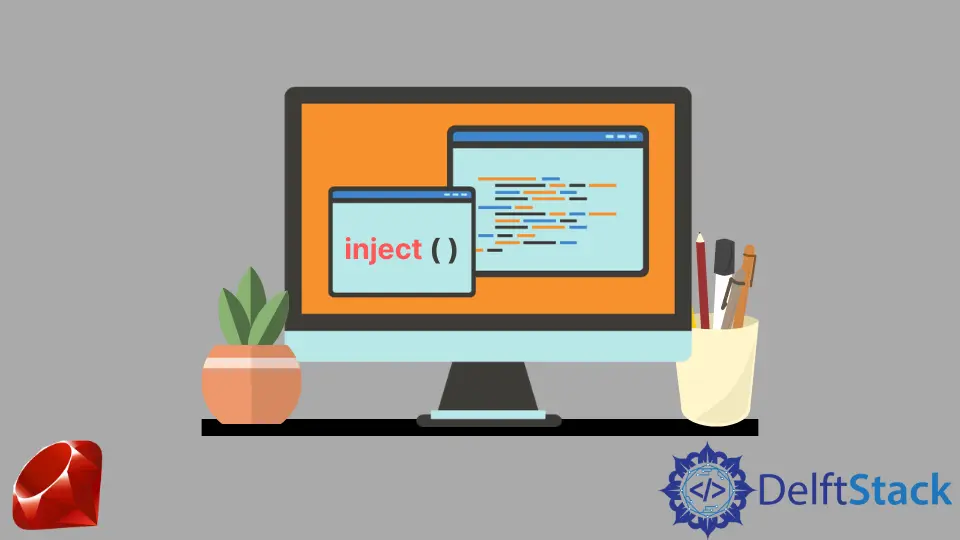
This article will demonstrate the different uses of the inject
method in Ruby.
Syntax of the Inject Method in Ruby
The inject
method takes a block of two arguments. The first argument is an accumulator, the running total of the expression being evaluated, and the second is the current array item.
Syntax:
inject(initial_value) { |_accumulator, _array_item| expression }
Sum an Array Using the Inject Method in Ruby
Example code:
numbers = [5, 6, 7, 8]
sum = numbers.inject(0) { |result, item| result + item }
puts sum
Output:
26
In the example above, the initial value is 0.
The accumulator is stored in the result
variable, which is updated on every iteration, and it’s the result of result + item
. The item
variable is the current array value.
Build a Hash from an Array Using the Inject Method in Ruby
Example code:
students = [['John', 'Grade 2'], ['Michael', 'Grade 3'], ['Stephen', 'Grade 4']]
students_hash = students.each_with_object({}) do |item, result|
result[item.first] = item.last
end
p students_hash
Output:
{"John"=>"Grade 2", "Michael"=>"Grade 3", "Stephen"=>"Grade 4"}
The above example transforms a nested array into a key-value hash, using the first element of each array item as the key and the second element as the value.
The initial accumulator value here is an empty hash {}
.
Filter an Array Using the Inject Method in Ruby
Example code:
numbers = [1, 2, 3, 4, 5, 6, 7]
even_numbers = numbers.each_with_object([]) do |element, result|
result << element if element.even?
end
p even_numbers
Output:
[2, 4, 6]
The above example builds a new array and populates it with the even numbers from the numbers
array.
The initial value here is an empty array []
.
inject
method is the reduce
method, and both can be used interchangeably.