How to Use Pattern Matching Operator in Ruby
- Understanding the Pattern Matching Operator
- Matching with Arrays
- Matching with Hashes
- Advanced Pattern Matching Techniques
- Conclusion
- FAQ
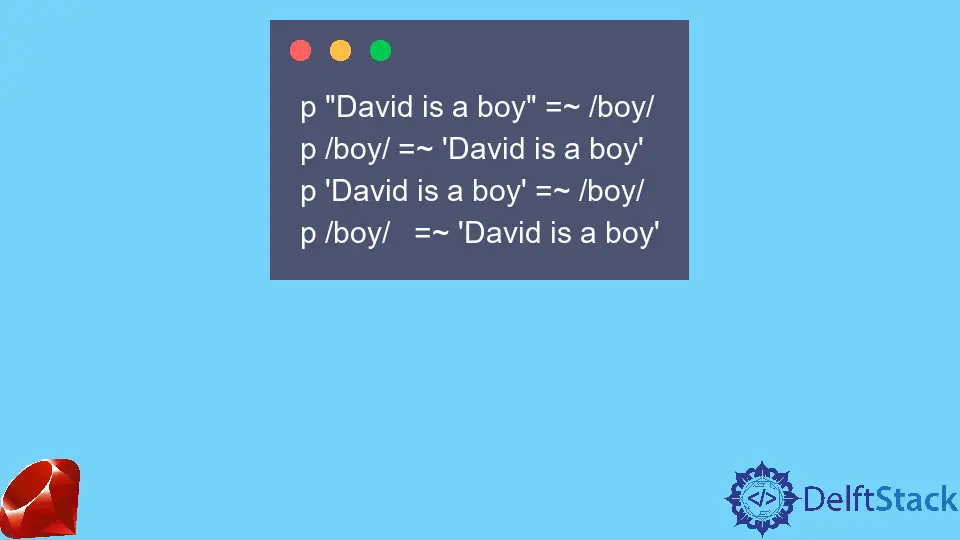
Pattern matching is an exciting feature in Ruby that offers a powerful way to destructure and analyze data. Introduced in Ruby 2.7, the pattern matching operator (===
) lets developers match patterns against values in a clear and concise manner. This feature not only simplifies code but also enhances readability, making it easier to handle complex data structures.
In this article, we’ll explore how to use the pattern matching operator in Ruby, providing clear examples and detailed explanations to help you grasp its functionality. Whether you’re a seasoned Rubyist or a beginner, understanding pattern matching can significantly improve your coding experience.
Understanding the Pattern Matching Operator
The pattern matching operator in Ruby is a versatile tool that allows you to match values against patterns, enabling you to destructure data with ease. This operator can be particularly useful when dealing with arrays, hashes, and other complex data types. By using the case
statement along with the pattern matching operator, you can create cleaner, more effective code.
Here’s a basic example to illustrate how the pattern matching operator works:
case [1, 2, 3]
in [a, b, c]
puts "Matched: #{a}, #{b}, #{c}"
end
In this example, we are using the case
statement to match an array. The pattern [a, b, c]
destructures the array into its components. When the case matches, it prints the values of a
, b
, and c
.
Output:
Matched: 1, 2, 3
This simple example demonstrates how easily you can unpack an array into individual variables. The pattern matching operator allows you to write less code while achieving the same functionality, making your Ruby scripts cleaner and more efficient.
Matching with Arrays
One of the most common uses of the pattern matching operator is with arrays. You can match specific elements or even patterns within arrays. Let’s look at a more complex example:
case [1, 2, 3]
in [1, *rest]
puts "First element is 1 and the rest are #{rest}"
end
In this case, we are using the splat operator (*
) to capture all remaining elements of the array after matching the first one. Here, if the first element is 1
, the rest of the elements will be stored in the variable rest
.
Output:
First element is 1 and the rest are [2, 3]
This example shows how the pattern matching operator can help you effectively manage array data. By using patterns, you can easily identify and extract the information you need without resorting to cumbersome indexing.
Matching with Hashes
Pattern matching isn’t limited to arrays; it works seamlessly with hashes as well. This capability allows you to destructure hash data and extract specific values. Here’s how you can do it:
case {name: "Alice", age: 30}
in {name: name, age: age}
puts "Name: #{name}, Age: #{age}"
end
In this example, we are matching a hash with keys name
and age
. When the case matches, it assigns the values to the respective variables.
Output:
Name: Alice, Age: 30
Using pattern matching with hashes allows for cleaner code when dealing with structured data. Instead of accessing values through keys, you can directly destructure them, making your code more readable and maintainable.
Advanced Pattern Matching Techniques
As you become more familiar with the pattern matching operator, you can explore advanced techniques like nested matching and guards. These features allow you to create more sophisticated patterns. For instance:
case {name: "Bob", age: 25}
in {name: name, age: age} if age > 20
puts "#{name} is an adult."
end
In this example, we are using a guard clause (if age > 20
) to ensure that the pattern only matches if the age is greater than 20. This adds an additional layer of logic to your pattern matching.
Output:
Bob is an adult.
This advanced technique demonstrates the flexibility of the pattern matching operator. You can combine various conditions and structures to create complex matching scenarios, enhancing the functionality of your Ruby applications.
Conclusion
The pattern matching operator in Ruby is a powerful feature that can significantly enhance your coding experience. By allowing you to destructure data easily and match patterns against values, it simplifies your code and improves readability. Whether you’re working with arrays, hashes, or more complex data types, pattern matching can help you write cleaner, more efficient Ruby code. As you practice and explore its capabilities, you’ll find that it opens up new possibilities for your programming projects.
FAQ
-
what is the pattern matching operator in Ruby?
The pattern matching operator in Ruby is===
, which allows you to match patterns against values, enabling easier destructuring of data. -
how does pattern matching improve code readability?
Pattern matching simplifies the code structure by reducing the need for explicit indexing or accessing keys, making it easier to understand and maintain. -
can I use pattern matching with nested structures?
Yes, pattern matching can be used with nested structures, allowing you to destructure complex data types effectively. -
what versions of Ruby support pattern matching?
Pattern matching was introduced in Ruby 2.7 and is available in all subsequent versions. -
are there performance benefits to using pattern matching?
While pattern matching can make code cleaner and easier to read, its performance benefits depend on the specific context and how it is implemented in your application.