The map Method in Ruby
- Understanding the Map Method
- Basic Usage of Map
- Using Map with Objects
- Combining Map with Other Enumerable Methods
- Conclusion
- FAQ
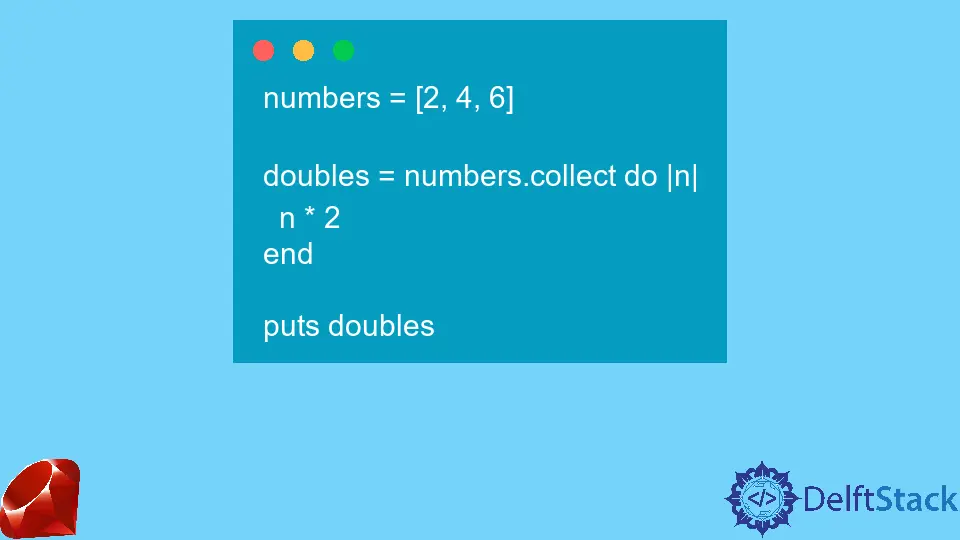
If you’re diving into Ruby, one of the first methods you’ll encounter is the map
method. It’s a powerful tool that transforms elements in an array, allowing you to manipulate data effortlessly. Whether you’re looking to change the format of your data, extract specific attributes from objects, or perform operations on each element, the map
method has got you covered.
In this article, we’ll explore what the map
method is, how to use it effectively, and provide you with plenty of examples to solidify your understanding. By the end, you’ll see just how versatile and handy this method can be in your Ruby programming toolkit.
Understanding the Map Method
The map
method is an enumerable method in Ruby that takes each element of an array (or any enumerable) and applies a block of code to it. The result is a new array containing the results of the block’s execution. This means you can transform each element in a collection without altering the original array.
Here’s a simple example to illustrate the concept:
numbers = [1, 2, 3, 4, 5]
squared_numbers = numbers.map { |number| number ** 2 }
Output:
[1, 4, 9, 16, 25]
In this example, we have an array of numbers. By using the map
method, we square each number and store the results in a new array called squared_numbers
. The original array remains unchanged, showcasing one of the core benefits of using map
.
Basic Usage of Map
The most straightforward way to use the map
method is to pass a block that performs some operation on each element of the array. Let’s look at a more detailed example:
fruits = ["apple", "banana", "cherry"]
capitalized_fruits = fruits.map { |fruit| fruit.capitalize }
Output:
["Apple", "Banana", "Cherry"]
In this scenario, we have an array of fruit names. By leveraging the map
method, we capitalize each name and store the results in a new array. The beauty of this method lies in its readability and efficiency. You can easily see what transformation is being applied to each element, making your code cleaner and more maintainable.
Using Map with Objects
The map
method is not limited to simple data types like integers or strings. You can also use it with objects. Suppose you have an array of user objects, and you want to extract their names. Here’s how you can do it:
class User
attr_accessor :name
def initialize(name)
@name = name
end
end
users = [User.new("Alice"), User.new("Bob"), User.new("Charlie")]
user_names = users.map { |user| user.name }
Output:
["Alice", "Bob", "Charlie"]
In this example, we define a User
class with a name
attribute. We then create an array of users and use the map
method to extract their names. This approach highlights the versatility of the map
method, as it can be used to work with complex data structures just as easily as with simple arrays.
Combining Map with Other Enumerable Methods
One of the strengths of Ruby is how its methods can be combined for more powerful data manipulations. You can chain the map
method with other enumerable methods like select
, reduce
, or reject
. For instance, if you want to filter out certain elements before mapping them, you can do it like this:
numbers = [1, 2, 3, 4, 5, 6]
even_squared_numbers = numbers.select { |number| number.even? }.map { |number| number ** 2 }
Output:
[4, 16, 36]
In this example, we first select the even numbers from the original array and then use the map
method to square those numbers. This chaining capability allows for concise and expressive code, making it easier to read and understand the transformations being applied.
Conclusion
The map
method in Ruby is a fundamental tool for any developer looking to manipulate arrays and other enumerable collections. Its simplicity and power allow you to transform data in a clean and efficient manner. Whether you’re working with simple arrays or complex objects, the map
method provides a clear syntax that enhances code readability. As you continue your journey with Ruby, mastering the map
method will undoubtedly make your programming more enjoyable and productive.
FAQ
-
What is the difference between map and collect in Ruby?
map and collect are synonymous in Ruby; both perform the same function of transforming elements in an array. -
Can I use map with hashes in Ruby?
Yes, you can use map with hashes, but you’ll need to convert the hash to an array first, typically using theto_a
method. -
Does the map method modify the original array?
No, the map method does not modify the original array; it returns a new array with the transformed elements. -
What happens if the block passed to map returns nil?
If the block returns nil for any element, that nil value will be included in the resulting array. -
Is map a method of the Array class only?
No, map is an enumerable method available to any object that includes the Enumerable module, including arrays and hashes.