How to Handle Exceptions Using Begin and Rescue in Ruby
- Understanding the Basics of Exception Handling
- Handling Multiple Exceptions
- Ensuring Cleanup with Ensure
- Raising Custom Exceptions
- Conclusion
- FAQ
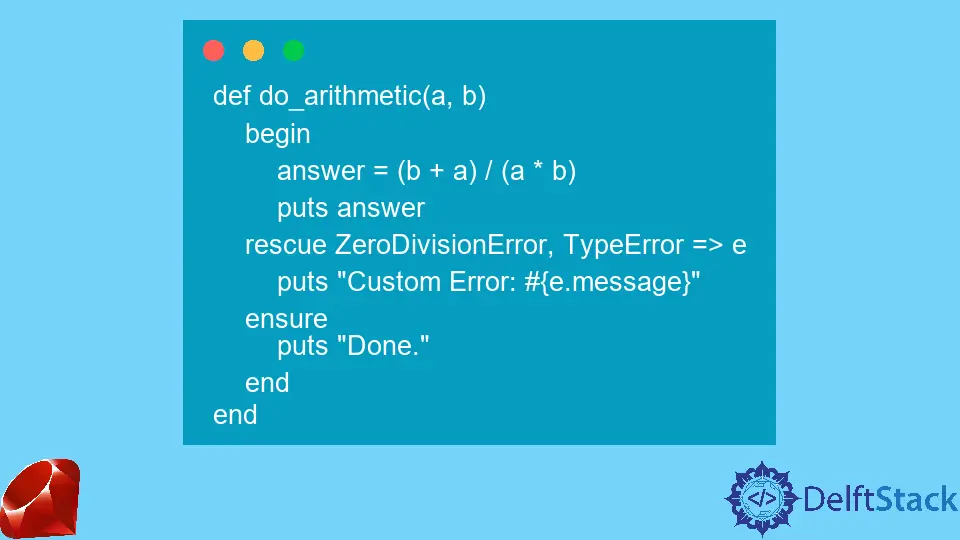
Handling exceptions is a vital part of programming, especially in Ruby, where robust error management can significantly enhance your application’s reliability. In Ruby, the begin
and rescue
keywords serve as the backbone of exception handling. They allow developers to gracefully manage errors and maintain control over the flow of their programs.
In this article, we will explore how to effectively use begin
and rescue
in Ruby to handle exceptions. We’ll provide code examples, detailed explanations, and practical tips to ensure you can implement these techniques in your own projects. Whether you’re a beginner or an experienced developer, mastering exception handling will elevate your Ruby skills and improve your code’s resilience.
Understanding the Basics of Exception Handling
In Ruby, exceptions are events that occur during the execution of a program that disrupt its normal flow. By using begin
and rescue
, you can catch these exceptions and handle them accordingly, preventing your program from crashing unexpectedly. The typical structure involves placing the code that may raise an exception inside a begin
block, followed by one or more rescue
blocks to manage different types of errors.
Here’s a simple example to illustrate this concept:
begin
puts "Enter a number:"
number = gets.chomp.to_i
result = 100 / number
puts "Result: #{result}"
rescue ZeroDivisionError
puts "You cannot divide by zero!"
rescue StandardError => e
puts "An error occurred: #{e.message}"
end
In this example, the program prompts the user to enter a number. If the user enters zero, a ZeroDivisionError
is raised, and the rescue block for that error is executed, displaying an appropriate message. If any other error occurs, the StandardError
rescue block captures it, allowing for a more general error handling approach.
The flexibility of begin
and rescue
allows developers to tailor their error handling to specific needs, making it a powerful feature of Ruby.
Handling Multiple Exceptions
One of the strengths of Ruby’s exception handling is the ability to manage multiple exceptions in a single begin
block. This allows you to specify different actions for different types of errors, enhancing the robustness of your application.
Consider the following example, where we handle both ZeroDivisionError
and ArgumentError
:
begin
puts "Enter a number:"
number = gets.chomp
result = 100 / Integer(number)
puts "Result: #{result}"
rescue ZeroDivisionError
puts "You cannot divide by zero!"
rescue ArgumentError
puts "Please enter a valid number!"
rescue StandardError => e
puts "An error occurred: #{e.message}"
end
In this code, we first attempt to convert the input to an integer. If the input is not a valid number, an ArgumentError
will be raised, triggering the respective rescue block. This structure allows us to provide specific feedback based on the type of error encountered, enhancing the user experience.
By handling multiple exceptions, you can create more informative and user-friendly applications. This approach not only helps in debugging but also ensures that users are aware of what went wrong, allowing them to correct their input without frustration.
Ensuring Cleanup with Ensure
Another essential feature of Ruby’s exception handling is the ensure
block. This block will execute regardless of whether an exception was raised or not, making it perfect for cleanup actions like closing files or releasing resources.
Here’s how you can use the ensure
keyword:
begin
file = File.open("example.txt", "r")
content = file.read
puts content
rescue Errno::ENOENT
puts "File not found!"
ensure
file.close if file
puts "File closed."
end
In this example, we attempt to open a file and read its content. If the file does not exist, an Errno::ENOENT
exception is raised, and we display an error message. Regardless of whether an error occurs, the ensure
block ensures that the file is closed properly, preventing resource leaks.
Using ensure
is a best practice in Ruby programming, as it guarantees that necessary cleanup actions are taken, maintaining the integrity of your application.
Raising Custom Exceptions
In addition to handling exceptions, Ruby allows you to create and raise custom exceptions. This feature is particularly useful when you want to signal specific error conditions in your application.
Here’s an example of defining and raising a custom exception:
class CustomError < StandardError; end
def risky_operation
raise CustomError, "This is a custom error message."
end
begin
risky_operation
rescue CustomError => e
puts "Caught a custom error: #{e.message}"
end
In this code, we define a custom exception called CustomError
. When the risky_operation
method is called, it raises this exception. The subsequent rescue block catches the custom exception and displays the error message.
Creating custom exceptions allows you to define application-specific error conditions, making your code more expressive and easier to understand. By using meaningful names and messages, you can enhance the clarity of your error handling.
Conclusion
Handling exceptions in Ruby using begin
and rescue
is a fundamental skill that every developer should master. By understanding how to manage multiple exceptions, utilize ensure
for cleanup, and create custom exceptions, you can build robust applications that gracefully handle errors. This not only improves the user experience but also enhances the maintainability of your code. As you continue to work with Ruby, remember the power of effective exception handling and incorporate these techniques into your programming toolkit.
FAQ
-
What is the purpose of the
begin
block in Ruby?
Thebegin
block is used to wrap code that may raise exceptions, allowing you to handle those exceptions usingrescue
. -
Can I handle multiple exceptions in a single
begin
block?
Yes, you can have multiplerescue
clauses within a singlebegin
block to handle different types of exceptions separately. -
What is the role of the
ensure
block in exception handling?
Theensure
block is executed after thebegin
andrescue
blocks, regardless of whether an exception was raised. It is useful for cleanup actions. -
How do I create a custom exception in Ruby?
You can create a custom exception by defining a new class that inherits fromStandardError
. You can then raise this exception using theraise
keyword. -
Is it necessary to handle exceptions in Ruby?
While it’s not strictly necessary, handling exceptions is crucial for creating reliable and user-friendly applications. It helps prevent crashes and provides meaningful feedback to users.