How to Square Array Element in Ruby
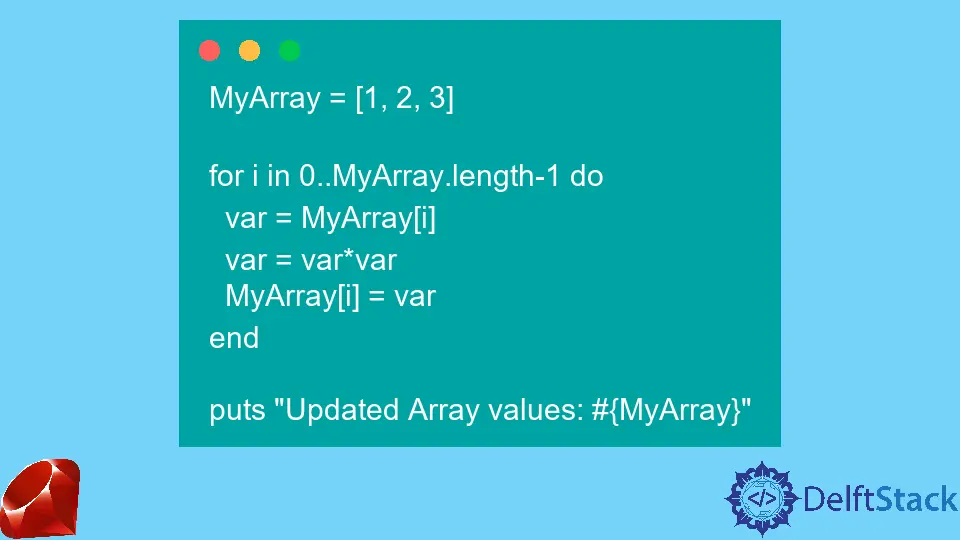
Square is a common mathematical operation that we perform on our regular days.
Now squaring a single variable is very easy, but it’s a bit complex when we need to square all the elements of an array. But we can do this in Ruby easily.
This tutorial will discuss how to square each element of an array. Also, we will look at some relevant examples to make the topic easier.
In this article, we will share two different ways to do this. First of all, we will use loops for this purpose, and then we will define a method inside the Array
class.
Method 1: Square Each Array Element Using a Loop
Below we will see how we can square each element of an array. For this purpose, we will use a for
loop.
The code will be like the one below.
MyArray = [1, 2, 3]
for i in 0..MyArray.length - 1 do
var = MyArray[i]
var *= var
MyArray[i] = var
end
puts "Updated Array values: #{MyArray}"
If you look at the code, you will see that we used a for
loop to visit each array element and square it. After running the above code example, you will have the below output.
Updated Array values: [1, 4, 9]
Method 2: Define a Method Inside the Array
Class
In our below example, we will see how we can square each element of an array. For this purpose, we will define a method in the Array
class.
The code will be like the one below.
class Array
def ValueSquare
map! { |var| var**2 }
end
end
puts [1, 2, 3].ValueSquare
In the above code, the method ValueSquare
will square each element of an array. Here the self.map!
will replace each array element with the result of squaring that element.
After running the above code example, you will have the below output.
1
4
9
Please note that all the code this article shares is written in Ruby.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn