yield in Ruby
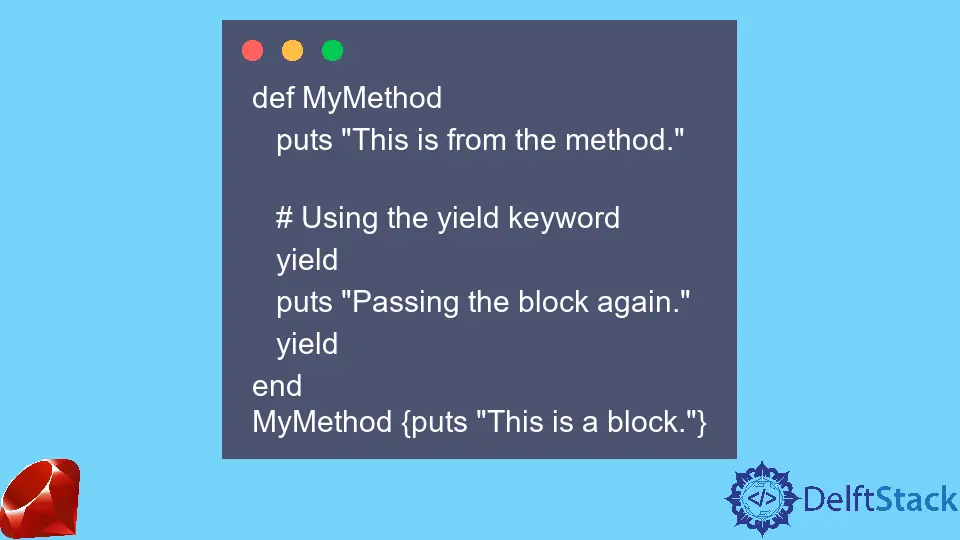
One of Ruby’s most exciting features is passing a block of code to a function. A built-in keyword in Ruby allows you to pass a block of codes to a function.
In this article, we will discuss the block
and yield
in Ruby. Also, we will look at some examples and explanations to make the topic easier.
A yield
is a built-in keyword that allows you to pass a block
. Now a block
is a code line enclosed by curly braces ({}
).
Let’s take a look at an example relevant to this topic.
Use the yield
Keyword in Ruby
The example below illustrates how we can use the keyword yield
in a Ruby program. Have a look at the below example code.
def MyMethod
puts 'This is from the method.'
# Using the yield keyword
yield
puts 'Passing the block again.'
yield
end
MyMethod { puts 'This is a block.' }
In the above example, we passed the block {puts "This is a block"}
to the function MyMethod
using the keyword yield
.
When you run the above codes, you will get the below output in your console.
This is from the method.
This is a block.
Passing the block again.
This is a block.
So the difference between the block
and yield
is that the block
is a collection of codes to perform specific tasks, and the yield
is a keyword that allows you to pass that block
in a function.
When a function gets a yield
keyword, it will run the block
that passed into it and then continue with the other lines.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn