YAML in Ruby
- Understanding YAML and Its Importance in Ruby
- Reading YAML Files in Ruby
- Writing YAML Files in Ruby
- Manipulating YAML Data in Ruby
- Conclusion
- FAQ
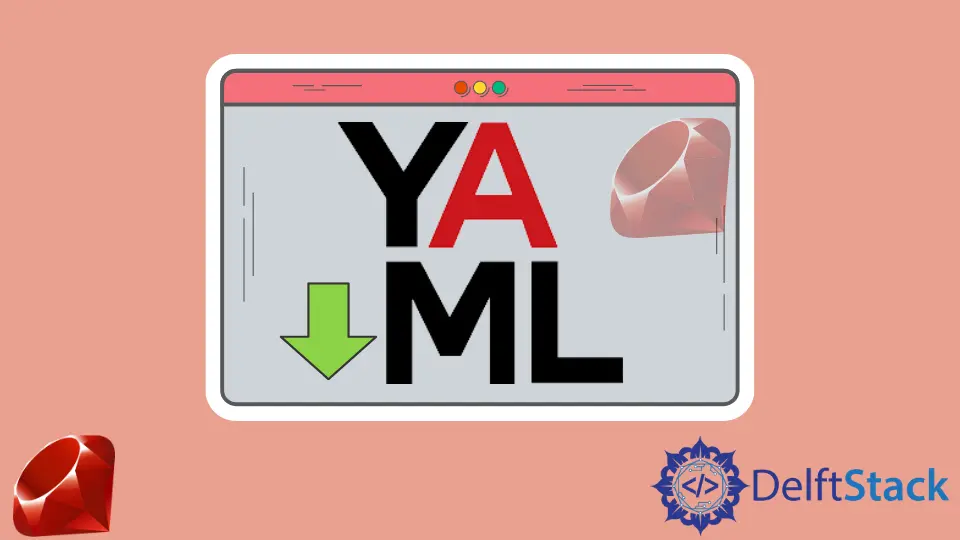
YAML (YAML Ain’t Markup Language) is a human-readable data serialization standard that is commonly used for configuration files and data exchange between languages. In the Ruby programming language, YAML plays a significant role in managing structured data.
This tutorial will guide you through the essentials of using YAML in Ruby, showcasing how to read, write, and manipulate YAML files effectively. Whether you’re a beginner or an experienced Ruby developer, understanding how to work with YAML can enhance your coding practices and improve your applications. Let’s dive in and explore how YAML integrates seamlessly with Ruby.
Understanding YAML and Its Importance in Ruby
Before we get into the nitty-gritty of YAML in Ruby, let’s clarify why YAML is so widely adopted. Its simplicity and readability make it an excellent choice for configuration files. Unlike XML or JSON, YAML uses indentation to represent structure, making it easier for humans to read and write.
In Ruby, the standard library includes a YAML module that allows you to easily handle YAML data. This module provides methods to parse YAML strings and files, as well as to generate YAML from Ruby objects. By leveraging these features, you can streamline your data handling processes in Ruby applications.
Reading YAML Files in Ruby
To read a YAML file in Ruby, you can use the YAML.load_file
method. This method loads the contents of a YAML file and converts it into a Ruby hash or array, depending on the structure of the YAML data.
Here’s a simple example demonstrating how to read a YAML file:
require 'yaml'
data = YAML.load_file('config.yml')
puts data.inspect
Output:
{"name"=>"John Doe", "age"=>30, "city"=>"New York"}
In this example, we first require the YAML library. Then, we call YAML.load_file
with the filename of the YAML file we want to read. The contents of the file are loaded into the data
variable, which is then printed out. The output shows the data as a Ruby hash, making it easy to access individual elements.
This method is particularly useful for loading configuration settings or any structured data that your Ruby application may require. By organizing your data in YAML format, you can easily modify it without changing your code.
Writing YAML Files in Ruby
Writing data to a YAML file in Ruby is equally straightforward. You can use the YAML.dump
method to convert Ruby objects into YAML format and then write them to a file. Here’s how you can do it:
require 'yaml'
data = {
name: 'Jane Doe',
age: 28,
city: 'Los Angeles'
}
File.open('output.yml', 'w') do |file|
file.write(YAML.dump(data))
end
Output:
name: Jane Doe
age: 28
city: Los Angeles
In this example, we create a Ruby hash named data
. We then open a file called output.yml
in write mode and use YAML.dump
to convert our hash into a YAML-formatted string. The write
method saves this string to the file. The output shows how the data is structured in YAML format, making it easy to read and understand.
This approach is particularly beneficial for exporting data from your Ruby application. Whether you’re saving user settings or application configurations, writing to a YAML file allows for easy data management.
Manipulating YAML Data in Ruby
Manipulating YAML data in Ruby involves reading the data, making changes, and then writing it back. This process is essential when you need to update configurations or user preferences dynamically. Let’s see how to do this step by step.
First, we read the existing YAML file, modify the data, and then write it back:
require 'yaml'
# Read the existing YAML file
data = YAML.load_file('config.yml')
# Modify the data
data['age'] = 31
data['city'] = 'San Francisco'
# Write the updated data back to the file
File.open('config.yml', 'w') do |file|
file.write(YAML.dump(data))
end
Output:
name: John Doe
age: 31
city: San Francisco
In this example, we start by loading the existing YAML file into the data
variable. We then update the age
and city
fields. Finally, we open the same file in write mode and save the modified data back using YAML.dump
. The output reflects the changes made to the original data.
This method is particularly useful for applications that need to adapt to user input or changing conditions. By manipulating YAML data in Ruby, you can create dynamic configurations that enhance the flexibility of your applications.
Conclusion
In this tutorial, we explored the essentials of using YAML in Ruby, covering how to read, write, and manipulate YAML files. With its human-readable format and ease of use, YAML is an invaluable tool for managing configurations and structured data in Ruby applications. By mastering these techniques, you can streamline your coding practices and enhance the functionality of your projects. Whether you’re building small scripts or large applications, understanding YAML can greatly improve your data handling capabilities.
FAQ
-
What is YAML?
YAML is a human-readable data serialization format commonly used for configuration files and data exchange between programming languages. -
How do I install the YAML library in Ruby?
The YAML library is included in Ruby’s standard library, so you don’t need to install it separately. Just require it in your script. -
Can I use YAML with other programming languages?
Yes, YAML is supported by many programming languages, including Python, Java, and JavaScript, making it a versatile choice for data serialization. -
What are the advantages of using YAML over JSON?
YAML is more readable and allows for complex data structures with less syntax clutter compared to JSON, making it easier for humans to read and write. -
How do I handle errors when reading YAML files in Ruby?
You can use exception handling (begin-rescue blocks) to manage errors when loading YAML files, ensuring your application can handle invalid data gracefully.