The %w Syntax Mean in Ruby
Stewart Nguyen
Feb 02, 2024
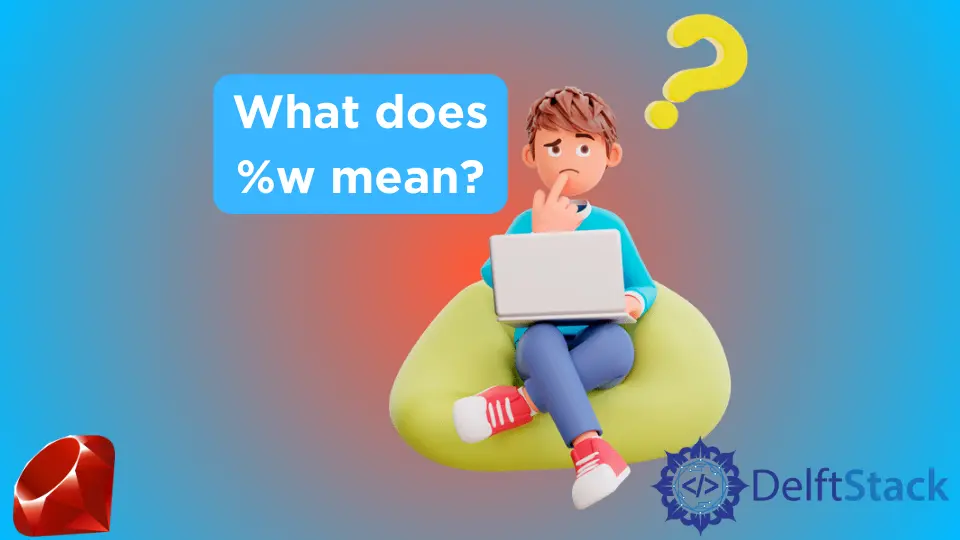
In Ruby, an array is a common data type. Strings, integers, hashes, symbols, and even other array objects can be stored in Ruby arrays.
We can use the following syntax to create an array of strings in ruby:
array = %w[one two three]
Output:
["one", "two", "three"]
It works, but it takes a lot of time and effort to type quotes and commas. Ruby provides us with a more elegant solution.
The %w
syntax is used to create an array of strings without the need for a comma or quotes between each element.
Each element will be treated as a string and should be separated by a space.
array = %w[1 two 3.4 [] {}]
Output:
["1", "two", "3.4", "[]", "{}"]