Ruby && vs and
Stewart Nguyen
Feb 02, 2024
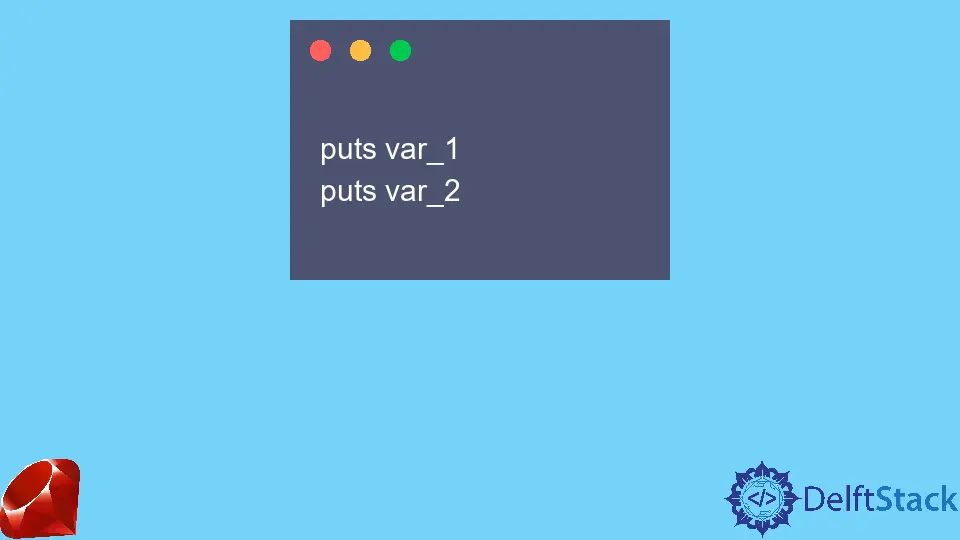
For boolean expressions or flow control, both &&
and and
can be used.
The difference between them is the order of precedence. Operator and
has lower precedence than =
whereas &&
has higher precedence than operator =
.
Consider these two boolean expressions.
puts var_1 = true && false
puts var_2 = true and false
Output:
false
true
Both expressions return false
, but var_1
and var_2
will have different values assigned to them.
var_1 = true && false
is equivalent to var_1 = (true && false)
, var_1
is equal to false
.
In contrast, var_2 = true and false
will be evaluated as (var_2 = true) and false
. This means that var_2
will eventually contain the value true
.
puts var_1
puts var_2
Output:
false
true
For this reason, never use and
with a ternary operator like this.
s = true and 2.even? ? 'even' : 'odd'
puts s
Output:
true
We may expect it prints even
, but instead, it returns true
.