Ruby to_sym Function
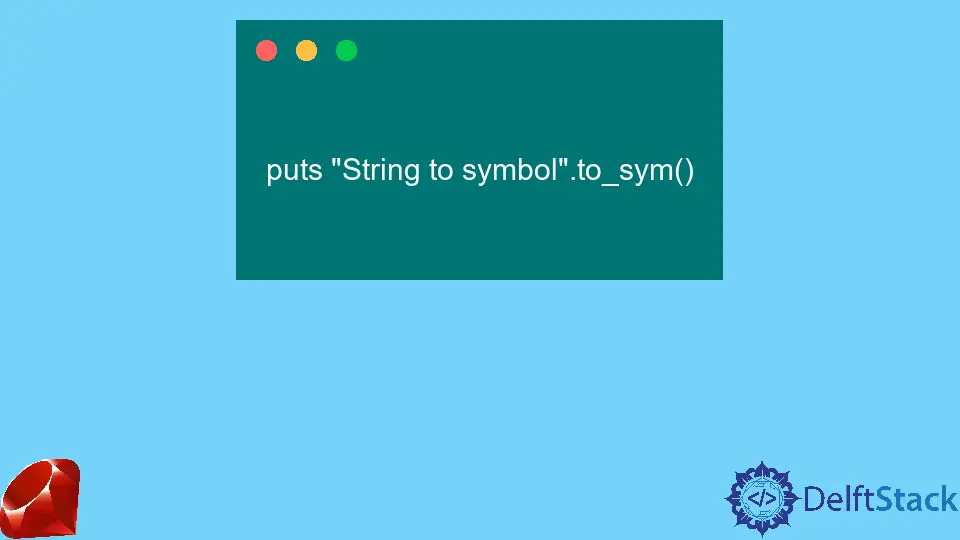
A symbol is a primitive data type that an instance, has a unique human-readable form. A symbol is mostly used as an identifier.
We also use symbols for various purposes.
In this article, we will discuss how to create a symbol in Ruby by using the to_sym()
function. Also, we will see an example relevant to the topic to make the topic easier.
The function to_sym()
is a built-in function in Ruby that allows you to generate a symbol of a variable or object. Let’s look at the below example.
Convert String to Symbol in Ruby
In our below example, we will demonstrate how we can generate a string symbol. Let’s look at the below example.
puts 'String to symbol'.to_sym
In the above example, we call the function to_sym()
for the string String to symbol
and print the output result.
After running the above example, you will get an output like the one below.
String to symbol
Here the string String to symbol
is now a symbol, and you can’t use it as a string.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn