super in Ruby
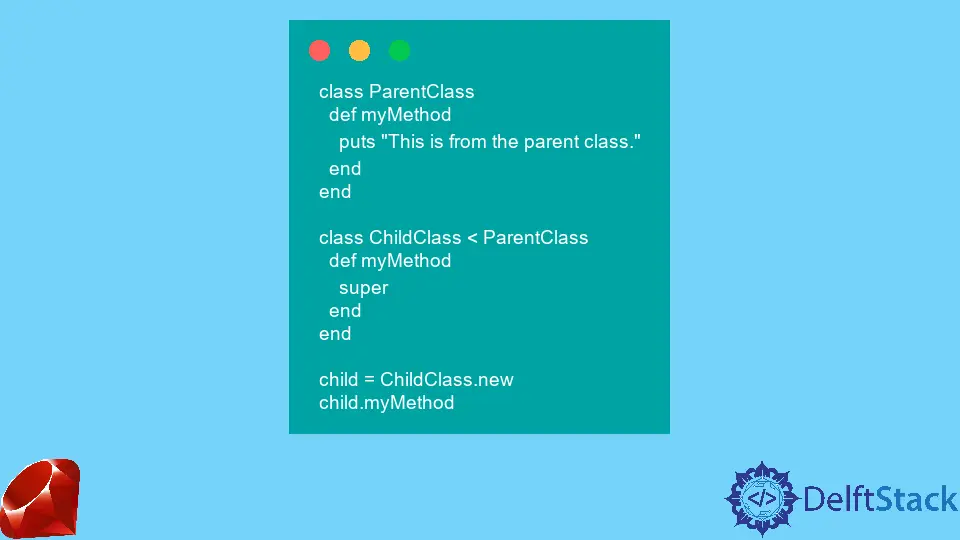
When developing programs on an object-oriented architecture, we must deal with many methods and classes. Sometimes we need to override or get the definition of a method already in a parent class.
In Ruby, it is easy to get the definition of a method in the parent class through the keyword super
.
In this article, we will see the use of the super
keyword in Ruby and look at an example to make the topic easier.
Use super
in Ruby
In the example below, we will illustrate the use of the super
keyword in Ruby. Let’s discuss the code below.
class ParentClass
def myMethod
puts 'This is from the parent class.'
end
end
class ChildClass < ParentClass
def myMethod
super
end
end
child = ChildClass.new
child.myMethod
In the above example, we have a ParentClass
with a method myMethod
. There is a ChildClass
that is the child class of ParentClass
with the same method, myMethod
.
Now, inside the method definition, we used the super
keyword to extract the definition of myMethod
from the parent class.
After that, we create an object of the ChildClass
and call the method myMethod
through these lines:
child = ChildClass.new
child.myMethod
After running the above code example, you will get the output below.
This is from the parent class.
You need to know a few things when working with the
super
keyword. The keywordsuper
can only be used inside a method, and the result it returns is from the parent method. Also, the child class should be inherited from the parent class.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn